Types of Exceptions
Introduction
Java is an object oriented programming language. The exception is object created at the time of exceptional/error condition which will be thrown from the program and halt normal execution of the program. Java exceptions object hierarchy is as below:
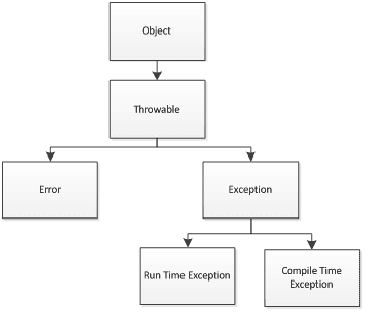
All exception types are subclasses of the built-in class Throwable. Thus, Throwable is at the top of the exception class hierarchy. Immediately below Throwable are two subclasses that partition exceptions into two distinct branches. One branch is headed by Exception. This classic used for exceptional conditions that user programs should catch. This is also the class that you will subclass to create your own custom exception types. There is an important subclass of Exception, called RuntimeException. Exceptions of this type are automatically defined for the programs that you write and include things such as division by zero and invalid array indexing.
The other branch is topped by Error, which defines exceptions that are not expected to be caught under normal circumstances by your program. Exceptions of type Error are used by the Java runtime system to indicate errors having to do with the runtime environment,itself. Stack overflow is an example of such an error. This chapter will not be dealing with exceptions of type Error, because these are typically created in response to catastrophic failures that cannot usually be handled by your program.
Java’s exceptions can be categorized into two types:
- Checked exceptions
- Unchecked exceptions
Generally, checked exceptions are subject to the catch or specify a requirement, which means they require catching or declaration. This requirement is optional for unchecked exceptions. Code that uses a checked exception will not compile if the catch or specify rule is not followed.
Unchecked exceptions come in two types:
- Errors
- Runtime exceptions
Checked Exceptions
Checked exceptions are the type that programmers should anticipate and from which programs should be able to recover. All Java exceptions are checked exceptions except those of the Error and RuntimeException classes and their subclasses.
A checked exception is an exception which the Java source code must deal with, either by catching it or declaring it to be thrown. Checked exceptions are generally caused by faults outside of the code itself - missing resources, networking errors, and problems with threads come to mind. These could include subclasses of FileNotFoundException, UnknownHostException, etc.
Popular Checked Exceptions:
Name | Description |
---|---|
IOException | While using file input/output stream related exception |
SQLException. | While executing queries on database related to SQL syntax |
DataAccessException | Exception related to accessing data/database |
ClassNotFoundException | Thrown when the JVM can’t find a class it needs, because of a command-line error, a classpath issue, or a missing .class file |
InstantiationException | Attempt to create an object of an abstract class or interface. |
Below example program of reading, file shows how checked exception should be handled. Below image shows compile time error due to checked exception (FileNotFoundException and IO Exception) related to file operation. IDE suggests either we need to enclose our code inside try-catch block or we can use throws keyword in the method declaration.
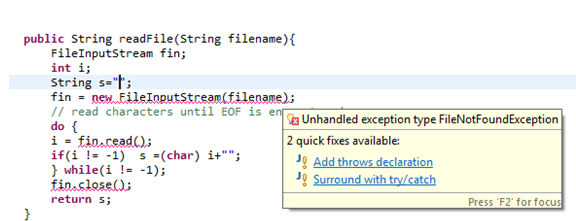
We will update the method declaration with throws keyword and calling method (main method) will have to handle this exception. I will explain file reading part in detail in file I/O tutorial, here we will concentrate more on exception part. While running the program we can encounter two types of problems (1) File is missing or not present, which we are trying to read (2) User does not have read permission on file or file is locked by some other user. As we are expecting two different type of exceptions we have to catch both exceptions or we can have one catch block which is catching super-class Exception. Below code shows multiple catch block syntax.
Java Code: Go to the editor
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class CheckedExceptionDemo {
public static void main(String[] args) {
//Below line calls readFile method and prints content of it
String filename="test.txt";
try {
String fileContent = new CheckedExceptionDemo().readFile(filename);
System.out.println(fileContent);
} catch (FileNotFoundException e) {
System.out.println("File:"+ filename+" is missing, Please check file name");
} catch (IOException e) {
System.out.println("File is not having permission to read, please check the permission");
}
}
public String readFile(String filename)throws FileNotFoundException, IOException{
FileInputStream fin;
int i;
String s="";
fin = new FileInputStream(filename);
// read characters until EOF is encountered
do {
i = fin.read();
if(i != -1) s =s+(char) i+"";
} while(i != -1);
fin.close();
return s;
}
}
Output: If test.txt is not found:
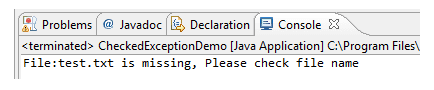
Running the program after creating test.txt file inside project root folder
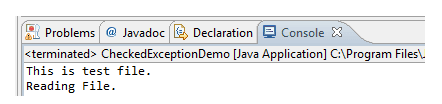
Unchecked Exceptions
Unchecked exceptions inherit from the Error class or the RuntimeException class. Many programmers feel that you should not handle these exceptions in your programs because they represent the type of errors from which programs cannot reasonably be expected to recover while the program is running.
When an unchecked exception is thrown, it is usually caused by a misuse of code - passing a null or otherwise incorrect argument.
Popular Unchecked Exceptions:
Name | Description |
---|---|
NullPointerException | Thrown when attempting to access an object with a reference variable whose current value is null |
ArrayIndexOutOfBound | Thrown when attempting to access an array with an invalid index value (either negative or beyond the length of the array) |
IllegalArgumentException. | Thrown when a method receives an argument formatted differently than the method expects. |
IllegalStateException | Thrown when the state of the environment doesn’t match the operation being attempted,e.g., using a Scanner that’s been closed. |
NumberFormatException | Thrown when a method that converts a String to a number receives a String that it cannot convert. |
ArithmaticException | Arithmetic error, such as divide-by-zero. |
We had seen sample program of runtime exception of divide by zero in last tutorial here we will see other program, which will take user age as input and grant access if age is more than 18 years. Here user input is expected in numeric form if user input is other alphabetic then our program will end in exception condition(InputMismatchException). This exception occurs at runtime. We can decide to handle it programmatically but it is not mandatory to handle. Runtime exceptions are good to handle using try-catch block and avoid error situation.
Java Code: Go to the editor
import java.util.Scanner;
public class RunTimeExceptionDemo {
public static void main(String[] args) {
//Reading user input
Scanner inputDevice = new Scanner(System.in);
System.out.print("Please enter your age- Numeric value: ");
int age = inputDevice.nextInt();
if (age>18){
System.out.println("You are authorized to view the page");
//Other business logic
}else {
System.out.println("You are not authorized to view page");
//Other code related to logout
}
}
}
Output:
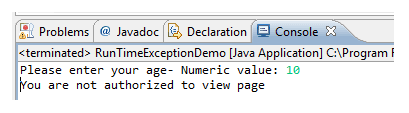
If User enters non-numeric value, program ends in error/exceptional condition.
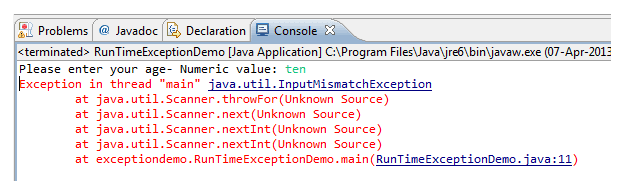
Summary:
- Loop condition/expression can be true always, which makes our loop infinite. This is bad programming practice as it might result in memory exception. Below statement is valid but not good to have in our program.
- In terms of Functionality Checked and Unchecked Exception are same.
- Checked Exception handling verified during compile time while Unchecked Exception is mostly programming errors
- JDK7 provides improved Exception handling code with catching multiple Exceptions in one catch block and reduce the amount of lines of code required for exception handling.
Java Code Editor:
Previous: Handling Exceptions
Next:Custom Exception