Java Collections Utility Class
Introduction
The Collections utility class consists exclusively of static methods that operate on or return collections. It contains polymorphic algorithms that operate on collections, "wrappers", which return a new collection backed by a specified collection,
Some useful method in Collections class:
Method Signature | Description |
Collections.sort(List myList) | Sort the myList (implementation of any List interface) provided an argument in natural ordering. |
Collections.sort(List, comparator c) | Sort the myList(implementation of any List interface) as per comparator c ordering (c class should implement comparator interface) |
Collections.shuffle(List myList) | Puts the elements of myList ((implementation of any List interface)in random order |
Collections.reverse(List myList) | Reverses the elements of myList ((implementation of any List interface) |
Collections.binarySearch(List mlist, T key) | Searches the mlist (implementation of any List interface) for the specified object using the binary search algorithm. |
Collections.copy(List dest, List src) | Copy the source List into dest List. |
Collections.frequency(Collection c, Object o) | Returns the number of elements in the specified collection class c (which implements Collection interface can be List, Set or Queue) equal to the specified object |
Collections.synchronizedCollection(Collection c) | Returns a synchronized (thread-safe) collection backed by the specified collection. |
Let's take the example of List sorting using Collection class. We can sort any Collection using “Collections” utility class. i.e.; ArrayList of Strings can be sorted alphabetically using this utility class. ArrayList class itself is not providing any methods to sort. We use Collections class static methods to do this. Below program shows use of reverse(), shuffle(), frequency() methods as well.
Java Code:
package utility;
import java.util.Collections;
import java.util.ArrayList;
import java.util.List;
public class CollectionsDemo {
public static void main(String[] args) {
List<String>student<String>List = new ArrayList();
studentList.add("Neeraj");
studentList.add("Mahesh");
studentList.add("Armaan");
studentList.add("Preeti");
studentList.add("Sanjay");
studentList.add("Neeraj");
studentList.add("Zahir");
System.out.println("Original List " + studentList);
Collections.sort(studentList);
System.out.println("Sorted alphabetically List " + studentList);
Collections.reverse(studentList);
System.out.println("Reverse List " + studentList);
Collections.shuffle(studentList);
System.out.println("Shuffled List " + studentList);
System.out.println("Checking occurance of Neeraj: "
+ Collections.frequency(studentList, "Neeraj"));
}
}
Output:
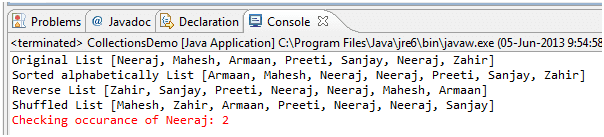
Using Collections class we can copy one type of collection to another type. Collections provide us copy method to copy all the elements from source to destination. Below program demonstrates the use of copy function. Here size of source collection and destination collection should be same else we will get following exception.

Java Code:Go to the editor
import java.util.Collections;
import java.util.*;
public class CopyListDemo {
public static void main(String[] args) {
List <Integer>myFirstList = new ArrayList<Integer>();
List <Integer> mySecondList = new ArrayList<Integer>();
myFirstList.add(10);
myFirstList.add(20);
myFirstList.add(20);
myFirstList.add(50);
myFirstList.add(70);
mySecondList.add(11);
mySecondList.add(120);
mySecondList.add(120);
mySecondList.add(150);
mySecondList.add(170);
System.out.println("First List-"+ myFirstList);
System.out.println("Second List-"+ mySecondList);
Collections.copy(mySecondList, myFirstList );
System.out.println("Second List After Copy-"+ mySecondList);
}
}
Output:
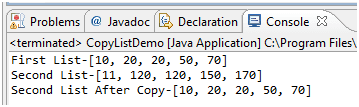
Java Code Editor:
Previous: Java Maps
Next: Java Defining, Instantiating and Starting Thread
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics