Print numbers with their squares and cubes for n lines
Print numbers with their squares and cubes for n lines
Write a C program to print a number, its square and cube, starting with 1 and printing n lines. Accept the number of lines (n, integer) from the user.
Pictorial Presentation:
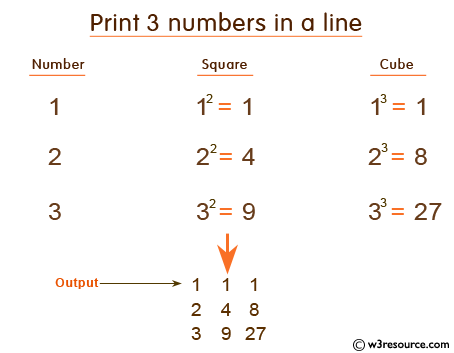
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int a, i, j = 1, x = 0;
// Prompt for user input
printf("Input number of lines: ");
scanf("%d", &a);
// Loop for each line
for(i = 1; i <= a; i++) {
// Print i, i squared, and i cubed
printf("%d %d %d\n", i, i*i, i*i*i);
}
return 0;
}
Sample Output:
Input number of lines: 5 1 1 1 2 4 8 3 9 27 4 16 64 5 25 125
Flowchart:
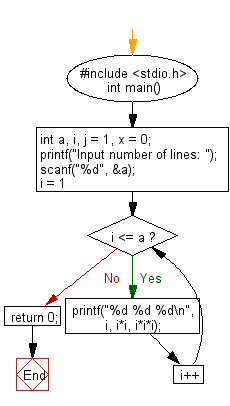
For more Practice: Solve these Related Problems:
- Write a C program to print numbers along with their squares, cubes, and fourth powers for n lines.
- Write a C program to display a table of numbers with their square roots and squares.
- Write a C program to print a list of numbers with their factorial values and corresponding power values.
- Write a C program to generate a table showing numbers, their exponential values (e^n), and their squared values.
C programming Code Editor:
Previous: Write a C program to print 3 numbers in a line, starting from 1 and print n lines. Accept number of lines (n, integer) from the user.
Next: Write a C program that reads two integers p and q, print p number of lines in a sequence of 1 to b in a line.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.