C Exercises: Check whether a number is a Strong Number or not
47. Strong Number Check
Write a C program to check whether a number is a Strong Number or not.
The problem requires writing a C program to determine if a number is a Strong Number. A Strong Number is one where the sum of the factorials of its digits equals the original number. The program should compute this sum and compare it to the original number to check if it is a Strong Number.
A Strong Number is a number where the sum of the factorials of its digits equals the number itself.
For example, 145 is a Strong Number:
- The digits are 1, 4, and 5.
- The factorial of 1 is 1! = 1.
- The factorial of 4 is 4! = 4 × 3 × 2 × 1 = 24.
- The factorial of 5 is 5! = 5 × 4 × 3 × 2 × 1 = 120.
- The sum of these factorials is 1 + 24 + 120 = 145.
Since the sum equals the original number, 145 is a Strong Number.
An example of a number that is not a Strong Number is 123.
To check if 123 is a Strong Number:
- Calculate the factorial of each digit:
- 1!=1
- 2!=2
- 3!=6
- Sum these factorials:
- 1+2+6=9
- Compare the sum to the original number:
- 9≠123
Since the sum of the factorials (9) does not equal the original number (123), 123 is not a Strong Number.
Visual Presentation:
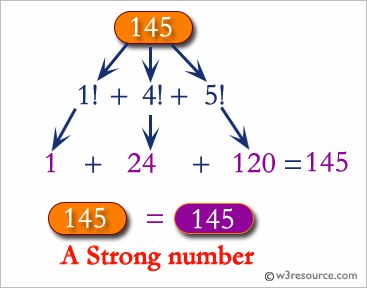
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, n, n1, s1 = 0, j; // Declare variables to store input and results.
long fact; // Declare a variable to store factorial.
printf("\n\nCheck whether a number is Strong Number or not:\n "); // Print a message.
printf("----------------------------------------------------\n"); // Print a separator.
printf("Input a number to check whether it is Strong number: "); // Prompt the user for input.
scanf("%d", &n); // Read the number from the user.
n1 = n; // Store the original number for comparison.
// Loop to process each digit of the number.
for (j = n; j > 0; j = j / 10)
{
fact = 1; // Initialize factorial.
// Loop to calculate factorial of a digit.
for (i = 1; i <= j % 10; i++)
{
fact = fact * i;
}
s1 = s1 + fact; // Accumulate factorial sum.
}
if (s1 == n1)
{
printf("\n%d is Strong number.\n\n", n1); // Print result if it's a strong number.
}
else
{
printf("\n%d is not Strong number.\n\n", n1); // Print result if it's not a strong number.
}
}
Output:
Check whether a number is Strong Number or not: ---------------------------------------------------- Input a number to check whether it is Strong number: 15 15 is not Strong number.
Flowchart:
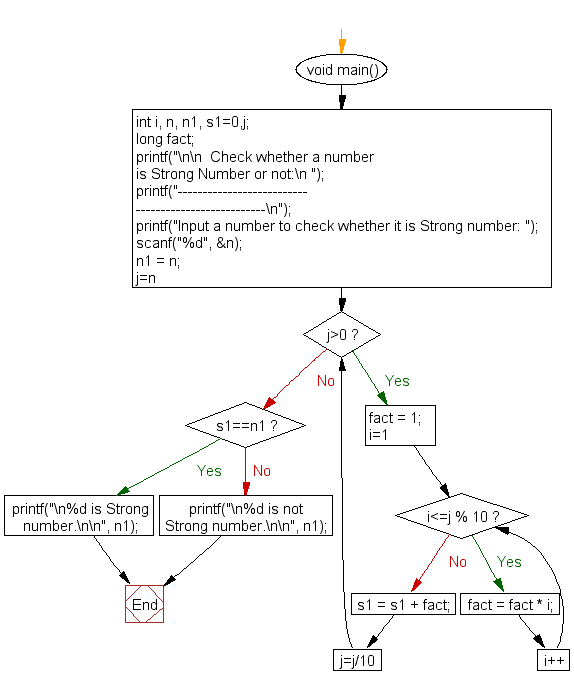
For more Practice: Solve these Related Problems:
- Write a C program to check if a number is a Strong number by computing the factorial of each digit recursively.
- Write a C program to verify a Strong number using iterative factorial calculation for each digit.
- Write a C program to check if a number is Strong and then print the factorial of each digit along with the sum.
- Write a C program to determine if a number is Strong and count the digits that meet a specific factorial threshold.
C Programming Code Editor:
Previous: Write a program in C to convert a binary number into a decimal number using math function.
Next: Write a C program to find Strong Numbers within a range of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.