C Exercises: Check a number is a Happy or not
13. Happy Number Check Variants
Write a program in C to determine whether a number is Happy or not.
Test DataInput a number: 13
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
#include <stdbool.h>
// Function to calculate the sum of squares of digits of a number
int SumOfSquNum(int givno)
{
int SumOfSqr = 0;
// Calculate the sum of squares of digits
while (givno)
{
SumOfSqr += (givno % 10) * (givno % 10); // Square each digit and add to the sum
givno /= 10; // Move to the next digit
}
return SumOfSqr; // Return the sum of squares of digits
}
// Function to check if a number is a Happy number
bool checkHappy(int chkhn)
{
int slno, fstno;
slno = fstno = chkhn;
// Detect a cycle or if the number reaches 1
do
{
slno = SumOfSquNum(slno); // Calculate the sum of squares of digits for slno
fstno = SumOfSquNum(SumOfSquNum(fstno)); // Calculate the sum of squares of digits twice for fstno
}
while (slno != fstno); // Continue until both numbers meet or 1 is reached
return (slno == 1); // If slno becomes 1, the number is a Happy number
}
// Main function
int main()
{
int hyno;
printf("\n\n Check whether a number is a Happy number or not: \n");
printf(" ---------------------------------------------------\n");
printf(" Input a number: ");
scanf("%d", &hyno); // Input a number
// Check if the input number is a Happy number and print the result
if (checkHappy(hyno))
printf("%d is a Happy number.\n", hyno);
else
printf("%d is not a Happy number.\n", hyno);
return 0;
}
Sample Output:
Input a number: 13 13 is a Happy number.
Visual Presentation:
Flowchart:
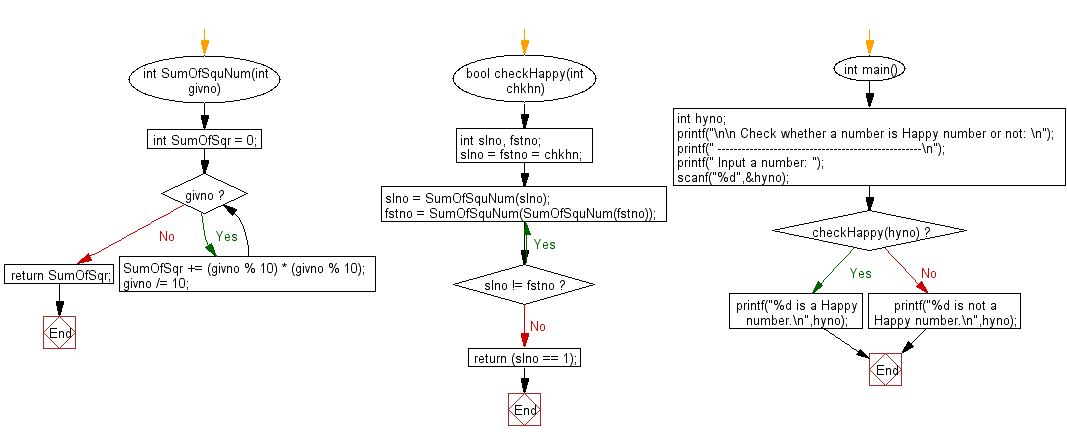
For more Practice: Solve these Related Problems:
- Write a C program to determine if a number is happy using cycle detection techniques.
- Write a C program to find happy numbers in a given range and display their iteration sequences.
- Write a C program to check happiness using a hash table to record previously seen sums.
- Write a C program to compare the happiness of a number in different numerical bases.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to display the first 10 catlan numbers.
Next: Write a program in C to find the happy numbers between 1 to 1000.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.