C Exercises: Find the largest element using Dynamic Memory Allocation
9. Largest Element Using Dynamic Memory Allocation
Write a program in C to find the largest element using Dynamic Memory Allocation.
Visual Presentation:
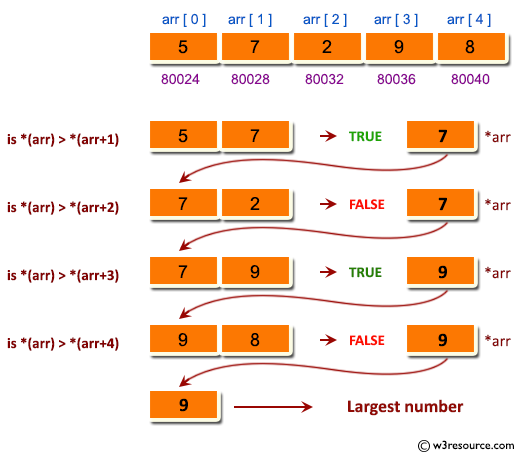
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
int main() {
int i, n;
float *element; // Pointer to float type to store elements
printf("\n\n Pointer : Find the largest element using Dynamic Memory Allocation :\n");
printf("-------------------------------------------------------------------------\n");
// Input the total number of elements
printf(" Input total number of elements (1 to 100): ");
scanf("%d", &n);
element = (float *)calloc(n, sizeof(float)); // Allocate memory for 'n' elements
if (element == NULL) {
printf(" No memory is allocated."); // If memory allocation fails
exit(0);
}
printf("\n");
// Input 'n' numbers and store them dynamically in the allocated memory
for (i = 0; i < n; ++i) {
printf(" Number %d: ", i + 1);
scanf("%f", element + i);
}
// Find the largest element among the 'n' elements
for (i = 1; i < n; ++i) {
if (*element < *(element + i)) {
*element = *(element + i); // Store the largest element in the first memory location
}
}
printf(" The Largest element is : %.2f \n\n", *element); // Display the largest element found
return 0;
}
Sample Output:
Pointer : Find the largest element using Dynamic Memory Allocation : ------------------------------------------------------------------------- Input total number of elements(1 to 100): 5 Number 1: 5 Number 2: 7 Number 3: 2 Number 4: 9 Number 5: 8 The Largest element is : 9.00
Flowchart:
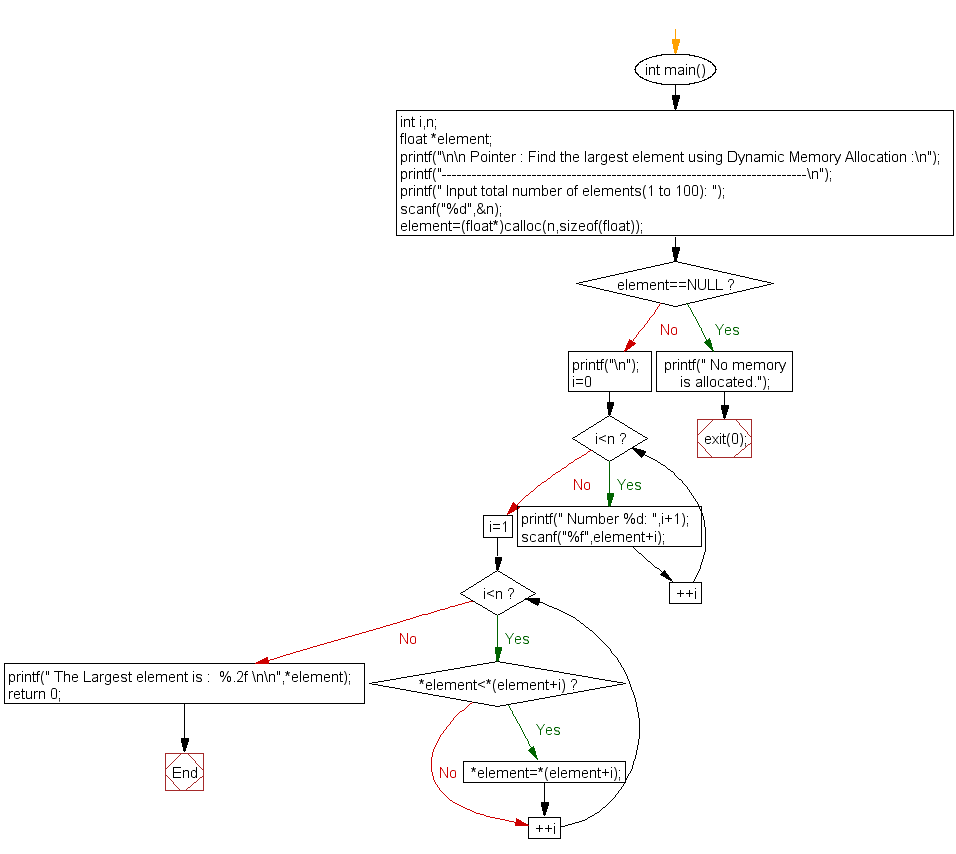
For more Practice: Solve these Related Problems:
- Write a C program to allocate memory for an array dynamically and find both the largest and smallest elements using pointers.
- Write a C program to find the largest element in a dynamically allocated array and then compute the average of the array elements.
- Write a C program to use dynamic memory allocation for an array, sort it using pointers, and then print the largest element.
- Write a C program to input n numbers dynamically and then find the largest element using recursion with pointers.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to print all permutations of a given string using pointers.
Next: Write a program in C to Calculate the length of the string using a pointer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.