C Exercises: Return the absolute value of a long integer
8. Absolute Value of a Long Integer
Write a C program to return the absolute value of a long integer.
Sample Solution:
C Code:
#include<stdio.h> // Include the standard input/output header file.
#include<stdlib.h> // Include the standard library header file.
int main () // Start of the main function.
{
long int x, y; // Declare two long integer variables 'x' and 'y'.
printf("\n Input 1st number (positive or negative) : "); // Prompt the user to input the first number.
scanf("%ld",&x); // Read the user's input and store it in 'x'.
printf(" Input 2nd number (positive or negative) : "); // Prompt the user to input the second number.
scanf("%ld",&y); // Read the user's input and store it in 'y'.
printf (" The absolute value of 1st number is : %ld\n",labs(x)); // Calculate and print the absolute value of 'x'.
printf (" The absolute value of 2nd number is : %ld\n\n",labs(y)); // Calculate and print the absolute value of 'y'.
return 0; // Return 0 to indicate successful execution of the program.
} // End of the main function.
Sample Output:
Input 1st number (positive or negative) : 25 Input 2nd number (positive or negative) : -125 The absolute value of 1st number is : 25 The absolute value of 2nd number is : 125
Flowchart:
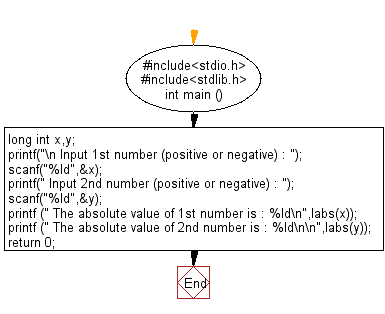
For more Practice: Solve these Related Problems:
- Write a C program to compute the absolute value of a long integer without using the abs() function.
- Write a C program to return the absolute value of a long integer and properly handle edge cases for minimum values.
- Write a C program to implement the absolute value function for long integers using bit manipulation.
- Write a C program to determine the absolute value of a long integer using conditional operators only.
C Programming Code Editor:
Previous: Write a C program to integral quotient and remainder of a division.
Next: Write a C program to get the environment string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.