C#: Display a number in rectangle of 3 columns wide and 5 rows tall using that digit
Rectangle Pattern with Number
Write a C# program that takes a number as input and displays a rectangle of 3 columns wide and 5 rows tall using that digit.
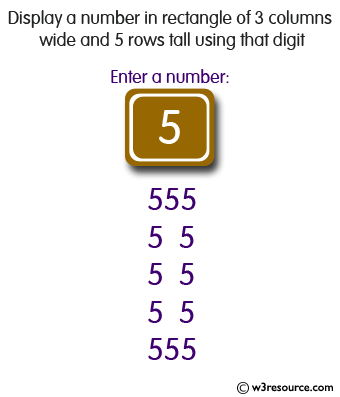
Sample Solution:
C# Sharp Code:
using System;
// This is the beginning of the Exercise13 class
public class Exercise13
{
// This is the main method where the program execution starts
public static void Main()
{
int x; // Variable to store the number entered by the user
// Prompting the user to enter a number
Console.Write("Enter a number: ");
// Reading the number entered by the user and converting it to an integer
x = Convert.ToInt32(Console.ReadLine());
// Printing the number in a specific pattern
Console.WriteLine("{0}{0}{0}", x); // Prints the number three times in the same line
Console.WriteLine("{0} {0}", x); // Prints the number twice with a space in between on each line
Console.WriteLine("{0} {0}", x); // Prints the number twice with a space in between on each line
Console.WriteLine("{0} {0}", x); // Prints the number twice with a space in between on each line
Console.WriteLine("{0}{0}{0}", x); // Prints the number three times in the same line
}
}
Sample Output:
Enter a number: 5 555 5 5 5 5 5 5 555
Flowchart:
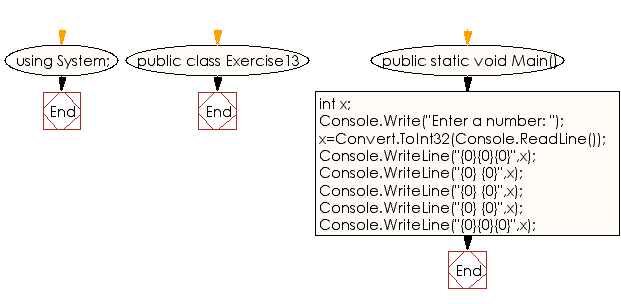
For more Practice: Solve these Related Problems:
- Write a C# program to display a hollow rectangle pattern using a digit entered by the user.
- Write a C# program to generate a rectangular numeric pattern that decreases the digit in each row.
- Write a C# program to print a digit in a rectangular frame with the border showing incrementing digits.
- Write a C# program to draw a rectangle with alternating digits in border and center rows.
Go to:
PREV : Repeat Number in Rows.
NEXT : Celsius to Kelvin and Fahrenheit.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.