C#: Check whether a triangle can be formed by given value
Write a C# Sharp program to check whether a triangle can be formed by the given angles value.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
public class Exercise15 // Declaration of the Exercise15 class
{
public static void Main() // Entry point of the program
{
int anga, angb, angc, sum; // Declaration of variables to store three angles of a triangle and their sum
Console.Write("\n\n"); // Printing new lines
Console.Write("Check whether a triangle can be formed by given value:\n"); // Displaying the purpose of the program
Console.Write("--------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
// Prompting user to input three angles of a triangle
Console.Write("Input angle1 of triangle: ");
anga = Convert.ToInt32(Console.ReadLine());
Console.Write("Input angle 2 of triangle: ");
angb = Convert.ToInt32(Console.ReadLine());
Console.Write("Input angle 3 of triangle: ");
angc = Convert.ToInt32(Console.ReadLine());
/* Calculate the sum of all angles of the triangle */
sum = anga + angb + angc; // Summing up the three angles
/* Check whether the sum is equal to 180 degrees to determine if it's a valid triangle */
if(sum == 180)
{
Console.Write("The triangle is valid.\n"); // Printing a message if the triangle is valid
}
else
{
Console.Write("The triangle is not valid.\n"); // Printing a message if the triangle is not valid
}
}
}
Sample Output:
Check whether a triangle can be formed by given value: -------------------------------------------------------- Input angle1 of triangle: 90 Input angle 2 of triangle: 45 Input angle 3 of triangle: 45 The triangle is valid.
Visual Presentation:
Flowchart:
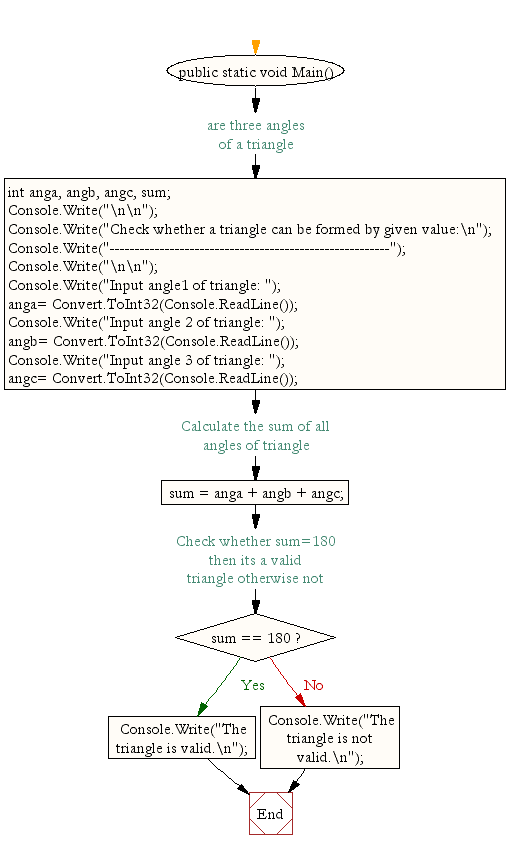
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to check whether a triangle is Equilateral, Isosceles or Scalene.
Next: Write a C# Sharp program to check whether an alphabet is a vowel or consonant.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.