C#: Display the DateTime value in the formatted output
Write a C# Sharp program to extract the Date property and display the DateTime value in the formatted output.
Sample Solution:-
C# Sharp Code:
using System;
public class Example1
{
// Main method, entry point of the program
public static void Main()
{
// Creating a DateTime object with specific date and time values
DateTime dt1 = new DateTime(2016, 6, 8, 11, 49, 0);
Console.WriteLine("Complete date: " + dt1.ToString()); // Display complete date and time
// Get the date portion (without time) from the DateTime object
DateTime dateOnly = dt1.Date;
// Displaying the date-only portion using "d" format specifier
Console.WriteLine("Short Date: " + dateOnly.ToString("d"));
// Displaying the date using 24-hour clock format
Console.WriteLine("Display date using 24-hour clock format:");
// Displaying the date and time in short format using "g" format specifier
Console.WriteLine(dateOnly.ToString("g"));
// Displaying the date and time in a custom format with 24-hour clock
Console.WriteLine(dateOnly.ToString("MM/dd/yyyy HH:mm"));
}
}
Sample Output:
Complete date: 6/8/2016 11:49:00 AM Short Date: 6/8/2016 Display date using 24-hour clock format: 6/8/2016 12:00 AM 06/08/2016 00:00
Flowchart :
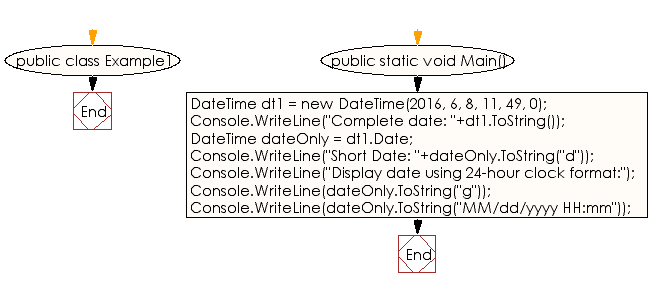
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: C# Sharp DateTime Exercises.
Next: Write a C# Sharp program to display the Day properties (year, month, day, hour, minute, second, millisecond etc.).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.