C#: Compute multiplication table of a given integer
Write a program in C# Sharp to display the multiplication table of a given integer.
Visual Presentation:
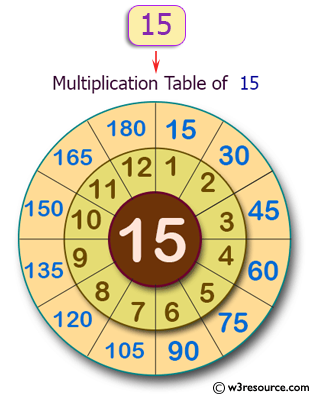
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise6 // Declaration of the Exercise6 class
{
public static void Main() // Main method, entry point of the program
{
int j, n; // Declaration of variables 'j' for iteration and 'n' to store the input number
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the multiplication table:\n"); // Displaying the purpose of the program
Console.Write("-----------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input the number (Table to be calculated) : "); // Prompting the user to input a number for the multiplication table
n = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
Console.Write("\n"); // Displaying a new line
for (j = 1; j <= 10; j++) // Loop to calculate and display the multiplication table of the entered number up to 10
{
Console.Write("{0} X {1} = {2} \n", n, j, n * j); // Displaying the multiplication table entry
}
}
}
Sample Output:
Display the multiplication table: ----------------------------------- Input the number (Table to be calculated) : 15 15 X 1 = 15 15 X 2 = 30 15 X 3 = 45 15 X 4 = 60 15 X 5 = 75 15 X 6 = 90 15 X 7 = 105 15 X 8 = 120 15 X 9 = 135 15 X 10 = 150
Flowchart:
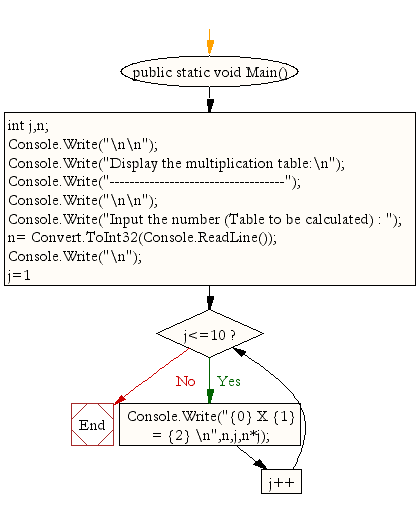
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the cube of the number upto given an integer.
Next: Write a program in C# Sharp to display the multiplication table vertically from 1 to n.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.