C#: Remove items from list by passing filters
Write a program in C# Sharp to remove items from list by passing filters.
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
// Define a class named LinqExercise19
class LinqExercise19
{
// Main method, the entry point of the program
static void Main(string[] args)
{
// Create a list of strings
List<string> listOfString = new List<string>();
// Add strings to the list
listOfString.Add("m");
listOfString.Add("n");
listOfString.Add("o");
listOfString.Add("p");
listOfString.Add("q");
// Display information about the program
Console.Write("\nLINQ : Remove items from list by passing filters : ");
Console.Write("\n--------------------------------------------------\n");
// Select all items in the list using LINQ and display them
var _result1 = from y in listOfString
select y;
Console.Write("Here is the list of items : \n");
foreach (var tchar in _result1)
{
Console.WriteLine("Char: {0} ", tchar);
}
// Remove all occurrences of "q" from the list using RemoveAll method and lambda expression
listOfString.RemoveAll(en => en == "q");
// Select and display all items in the list after removing "q"
var _result = from z in listOfString
select z;
Console.Write("\nHere is the list after removing item 'q' from the list : \n");
foreach (var rChar in _result)
{
Console.WriteLine("Char: {0} ", rChar);
}
Console.ReadLine(); // Wait for user input before closing the program
}
}
Sample Output:
LINQ : Remove items from list by passing filters : -------------------------------------------------- Here is the list of items : Char: m Char: n Char: o Char: p Char: q Here is the list after removing item 'q' from the list : Char: m Char: n Char: o Char: p
Visual Presentation:
Flowchart:
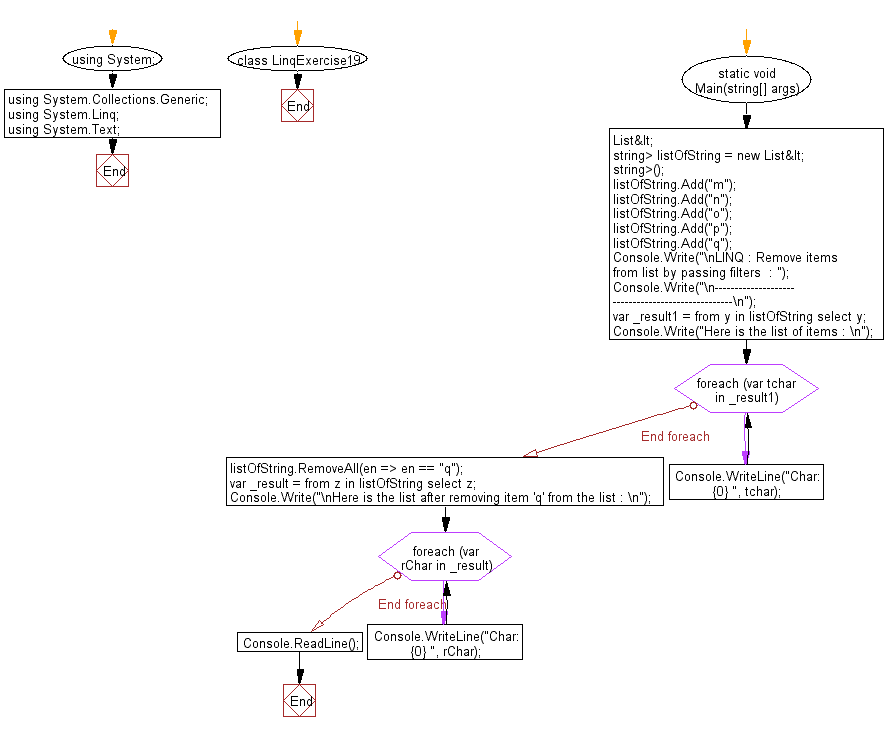
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to Remove Items from List by creating an object internally by filtering.
Next: Write a program in C# Sharp to Remove Items from List by passing the item index.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.