C#: Remove range of items from list by passing start index and number of elements to delete
Write a program in C# Sharp to remove a range of items from a list by passing the start index and number of elements to remove.
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
class LinqExercise21
{
static void Main(string[] args)
{
// Initialize a list of strings
List<string> listOfString = new List<string>();
listOfString.Add("m");
listOfString.Add("n");
listOfString.Add("o");
listOfString.Add("p");
listOfString.Add("q");
// Display a message indicating the purpose of the following output
Console.Write("\nLINQ : Remove range of items from list by passing start index and number of elements to delete : ");
Console.Write("\n------------------------------------------------------------------------------------------------\n");
// Using LINQ to select all items from the list
var _result1 = from y in listOfString
select y;
// Display the initial list items
Console.Write("Here is the list of items : \n");
foreach (var tchar in _result1)
{
Console.WriteLine("Char: {0} ", tchar);
}
// Remove a range of items from the list starting from index 1 and deleting 3 elements
listOfString.RemoveRange(1, 3);
// Using LINQ to select all items after removing a range of items from the list
var _result = from z in listOfString
select z;
Console.Write("\nHere is the list after removing the three items starting from the item index 1 from the list : \n");
// Display the updated list items
foreach (var rChar in _result)
{
Console.WriteLine("Char: {0} ", rChar);
}
Console.ReadLine(); // Wait for user input before exiting
}
}
Sample Output:
LINQ : Remove range of items from list by passing start index and number of elements to delete : ------------------------------------------------------------------------------------------------ Here is the list of items : Char: m Char: n Char: o Char: p Char: q Here is the list after removing the three items starting from the item index 1 from the list : Char: m Char: q
Visual Presentation:
Flowchart:
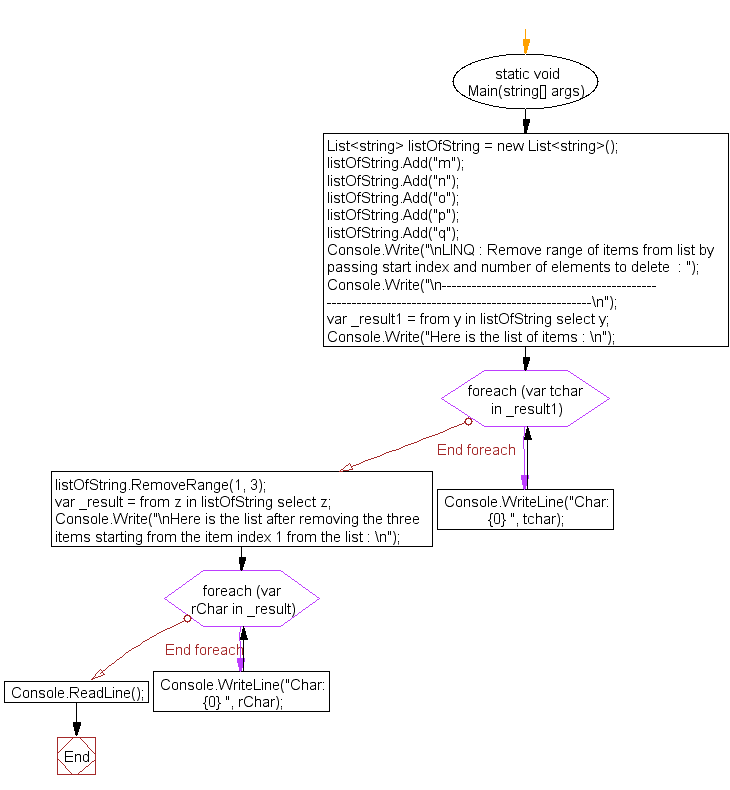
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to Remove Items from List by passing the item index.
Next: Write a program in C# Sharp to find the strings for a specific minimum length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.