Java: Iterate through all elements in a tree set
2. Iterate TreeSet Elements
Write a Java program to iterate through all elements in a tree set.
Sample Solution:
Java Code:
import java.util.TreeSet;
public class Exercise2 {
public static void main(String[] args) {
TreeSet<String> tree_set = new TreeSet<String>();
tree_set.add("Red");
tree_set.add("Green");
tree_set.add("Orange");
tree_set.add("White");
tree_set.add("Black");
// Print the tree list
for (String element : tree_set) {
System.out.println(element);
}
}
}
Sample Output:
Black Green Orange Red White
Flowchart:
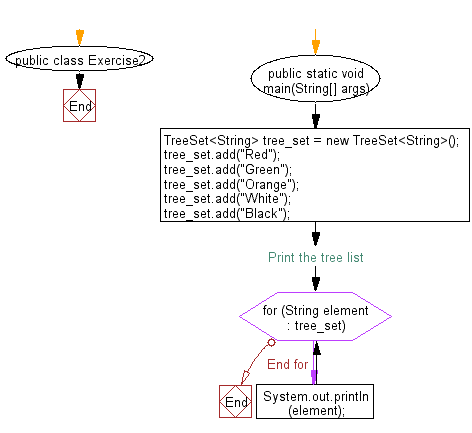
For more Practice: Solve these Related Problems:
- Write a Java program to iterate over a TreeSet using an Iterator and print each element along with its hash code.
- Write a Java program to iterate through a TreeSet using a for-each loop and conditionally print only those elements that start with a vowel.
- Write a Java program to traverse a TreeSet using Java 8 streams and print each element in uppercase.
- Write a Java program to iterate over a TreeSet in reverse order by converting it to a descending set and then printing each element.
Go to:
PREV : Create and Print TreeSet.
NEXT : Add Elements to Another TreeSet.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.