JavaScript: Fill an array with values on supplied bounds
JavaScript Array: Exercise-29 with Solution
Fill Array with Values
Write a JavaScript function to fill an array with values (numeric, string with one character) within supplied bounds.
Test Data:
console.log(num_string_range('a', "z", 2));
["a", "c", "e", "g", "i", "k", "m", "o", "q", "s", "u", "w", "y"]
Sample Solution:
JavaScript Code:
// Function to generate a range of numbers or characters based on start, end, and step
function num_string_range(start, end, step) {
// Initialize an empty array 'range' to store the generated range
var range = [];
// Check for invalid step value or undefined start/end values
if ((step === 0) || (typeof start === "undefined" || typeof end === "undefined") || (typeof start !== typeof end))
return false;
// Adjust the step direction if end is less than start
if (end < start) {
step = -step;
}
// Check if the start value is a number
if (typeof start === "number") {
// Generate a range of numbers
while (step > 0 ? end >= start : end <= start) {
range.push(start);
start += step;
}
}
// Check if the start value is a string
else if (typeof start === "string") {
// Check if both start and end are single characters
if (start.length !== 1 || end.length !== 1) {
throw TypeError("Strings with one character are supported.");
}
// Convert characters to their ASCII codes
start = start.charCodeAt(0);
end = end.charCodeAt(0);
// Generate a range of characters
while (step > 0 ? end >= start : end <= start) {
range.push(String.fromCharCode(start));
start += step;
}
}
// Throw an error for unsupported types
else {
throw TypeError("Only string and number are supported");
}
// Return the generated range
return range;
}
// Output the result of the num_string_range function with sample inputs
console.log(num_string_range('a', "z", 2));
console.log(num_string_range("Z", "A", 2));
console.log(num_string_range(0, -5, 1));
console.log(num_string_range(0, 25, 5));
console.log(num_string_range(20, 5, 5));
Output:
["a","c","e","g","i","k","m","o","q","s","u","w","y"] ["Z","X","V","T","R","P","N","L","J","H","F","D","B"] [0,-1,-2,-3,-4,-5] [0,5,10,15,20,25] [20,15,10,5]
Flowchart:
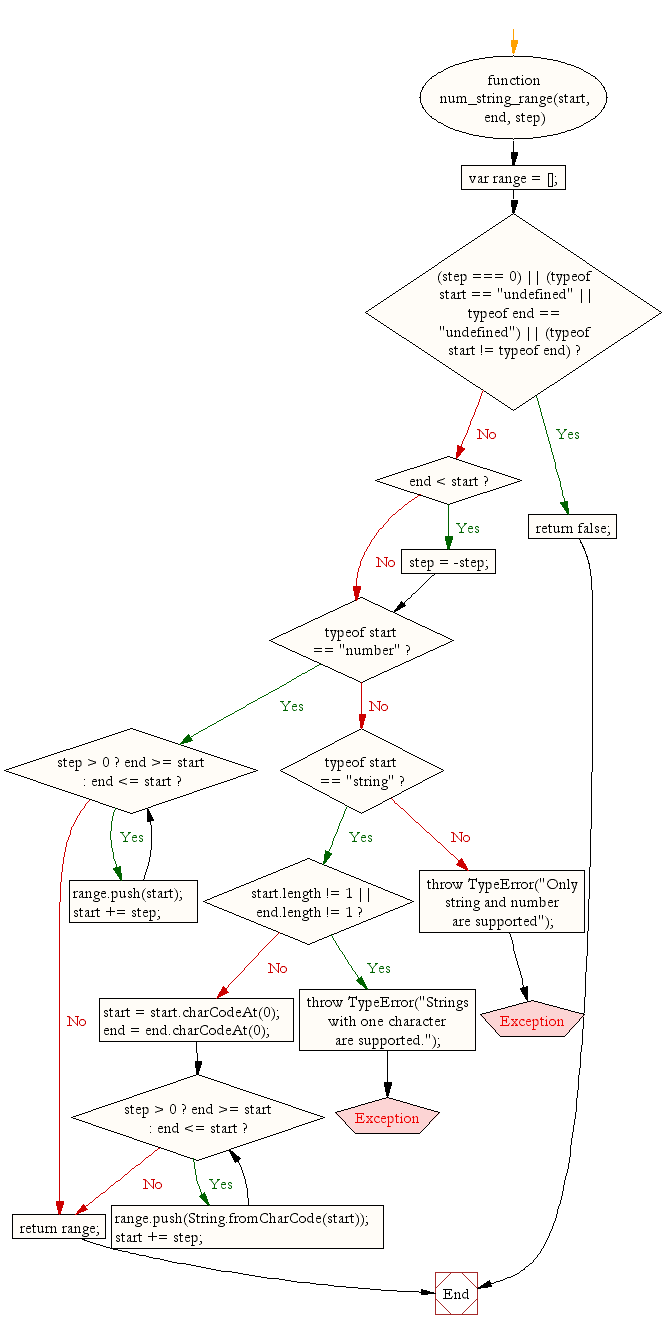
ES6 Version:
// Function to generate a range of numbers or characters based on start, end, and step
const num_string_range = (start, end, step) => {
// Initialize an empty array 'range' to store the generated range
const range = [];
// Check for invalid step value or undefined start/end values
if ((step === 0) || (typeof start === "undefined" || typeof end === "undefined") || (typeof start !== typeof end))
return false;
// Adjust the step direction if end is less than start
if (end < start) {
step = -step;
}
// Check if the start value is a number
if (typeof start === "number") {
// Generate a range of numbers
while (step > 0 ? end >= start : end <= start) {
range.push(start);
start += step;
}
}
// Check if the start value is a string
else if (typeof start === "string") {
// Check if both start and end are single characters
if (start.length !== 1 || end.length !== 1) {
throw TypeError("Strings with one character are supported.");
}
// Convert characters to their ASCII codes
start = start.charCodeAt(0);
end = end.charCodeAt(0);
// Generate a range of characters
while (step > 0 ? end >= start : end <= start) {
range.push(String.fromCharCode(start));
start += step;
}
}
// Throw an error for unsupported types
else {
throw TypeError("Only string and number are supported");
}
// Return the generated range
return range;
};
// Output the result of the num_string_range function with sample inputs
console.log(num_string_range('a', "z", 2));
console.log(num_string_range("Z", "A", 2));
console.log(num_string_range(0, -5, 1));
console.log(num_string_range(0, 25, 5));
console.log(num_string_range(20, 5, 5));
Live Demo:
See the Pen JavaScript - Fill an array with values on supplied bounds - array-ex- 29 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that creates an array of a specified length filled with a given numeric or string value using a loop.
- Write a JavaScript function that uses Array.from() to generate an array filled with a default value.
- Write a JavaScript function that leverages the fill() method to create a pre-filled array and returns it.
- Write a JavaScript function that validates the provided length and default value before creating the array.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to find the longest common starting substring in a set of strings.
Next: Write a JavaScript function to remove a specific element from an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.