JavaScript: Replace every character in a given string with the character following it in the alphabet
JavaScript Basic: Exercise-49 with Solution
Replace Each Character with Next Alphabet Letter
Write a JavaScript program to replace every character in a given string with the character following it in the alphabet. Convert vowels to uppercase.
This JavaScript program replaces each character in a string with the next character in the alphabet, and converts vowels to uppercase. It iterates through the string, modifies each character accordingly, and then constructs the new modified string.
Visual Presentation:
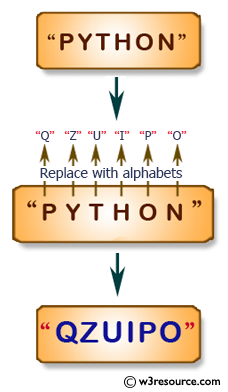
Sample Solution:
JavaScript Code:
// Define a function named LetterChanges with parameter text
function LetterChanges(text) {
// Initialize an array by splitting the input text into individual characters
var s = text.split('');
// Iterate through each character in the array
for (var i = 0; i < s.length; i++) {
// Caesar cipher: Shift each character by one position in the alphabet
switch(s[i]) {
case ' ':
break;
case 'z':
s[i] = 'a';
break;
case 'Z':
s[i] = 'A';
break;
default:
s[i] = String.fromCharCode(1 + s[i].charCodeAt(0));
}
// Convert vowels to uppercase
switch(s[i]) {
case 'a': case 'e': case 'i': case 'o': case 'u':
s[i] = s[i].toUpperCase();
}
}
// Join the modified characters back into a string and return the result
return s.join('');
}
// Log the result of calling LetterChanges with the argument "PYTHON" to the console
console.log(LetterChanges("PYTHON"));
// Log the result of calling LetterChanges with the argument "W3R" to the console
console.log(LetterChanges("W3R"));
// Log the result of calling LetterChanges with the argument "php" to the console
console.log(LetterChanges("php"));
Output:
QZUIPO X4S qIq
Live Demo:
See the Pen JavaScript - Replace every character in a given string with the character following it in the alphabet - basic-ex-49 by w3resource (@w3resource) on CodePen.
Flowchart:
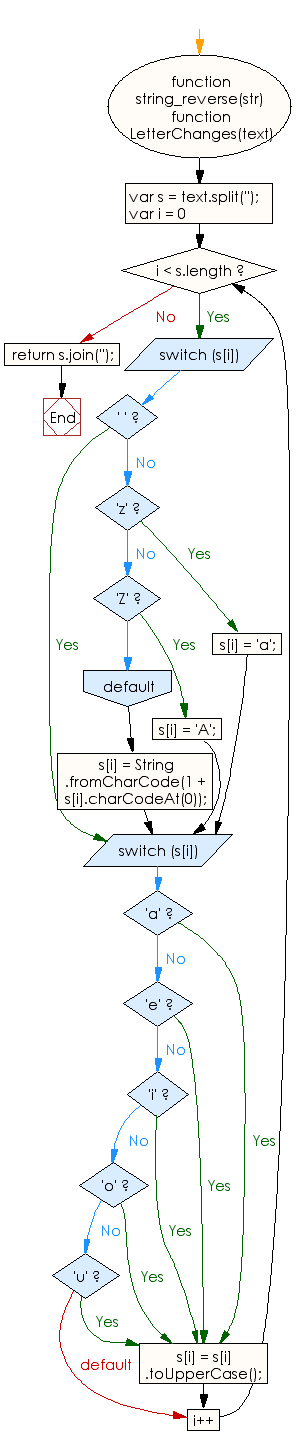
ES6 Version:
// Define a function named LetterChanges with parameter text
const LetterChanges = (text) => {
// Split the input text into an array of characters
let s = text.split('');
// Iterate through each character in the array
for (let i = 0; i < s.length; i++) {
// Caesar cipher
switch (s[i]) {
case ' ':
break;
case 'z':
s[i] = 'a';
break;
case 'Z':
s[i] = 'A';
break;
default:
s[i] = String.fromCharCode(1 + s[i].charCodeAt(0));
}
// Upper-case vowels
switch (s[i]) {
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
s[i] = s[i].toUpperCase();
}
}
// Join the modified array back into a string and return it
return s.join('');
};
// Log the result of calling LetterChanges with the argument "PYTHON" to the console
console.log(LetterChanges("PYTHON"));
// Log the result of calling LetterChanges with the argument "W3R" to the console
console.log(LetterChanges("W3R"));
// Log the result of calling LetterChanges with the argument "php" to the console
console.log(LetterChanges("php"));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that replaces every letter in a given string with the next letter in the alphabet, wrapping from 'z' to 'a'.
- Write a JavaScript program that shifts each character in a string forward by 2 positions in the alphabet.
- Write a JavaScript program that replaces each alphabet character in a string with the letter two positions ahead, leaving non-alphabet characters unchanged.
Go to:
PREV : Reverse a Given String.
NEXT : Capitalize First Letter of Each Word in String.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.