JavaScript: Get time differences in days between two dates
JavaScript Datetime: Exercise-46 with Solution
Time Diff in Days
Write a JavaScript function to get time differences in days between two dates.
Test Data:
dt1 = new Date("October 13, 2014 08:11:00");
dt2 = new Date("October 19, 2014 11:13:00");
console.log(diff_days(dt1, dt2));
6
Sample Solution:
JavaScript Code:
// Define a function diff_days that calculates the difference in days between two Date objects dt2 and dt1
function diff_days(dt2, dt1)
{
// Calculate the difference in milliseconds between dt2 and dt1
var diff = (dt2.getTime() - dt1.getTime()) / 1000;
// Convert the difference to days by dividing it by the number of seconds in a day (86400)
diff /= (60 * 60 * 24);
// Return the absolute value of the rounded difference in days
return Math.abs(Math.round(diff));
}
// Create two Date objects representing November 2, 2014, and November 6, 2014
dt1 = new Date(2014,10,2);
dt2 = new Date(2014,10,6);
// Output the difference in days between the two Date objects
console.log(diff_days(dt1, dt2));
// Create two Date objects representing October 13, 2014, at 08:11:00 and October 19, 2014, at 11:13:00
dt1 = new Date("October 13, 2014 08:11:00");
dt2 = new Date("October 19, 2014 11:13:00");
// Output the difference in days between the two Date objects
console.log(diff_days(dt1, dt2));
Output:
4 6
Explanation:
In the exercise above,
- The "diff_days()" function takes two Date objects, 'dt2' and 'dt1', as parameters.
- Inside the function, it calculates the difference in milliseconds between 'dt2' and 'dt1' using the "getTime()" method.
- It then converts the difference from milliseconds to days by dividing it by the number of milliseconds in a day, which is calculated as (60 60 24).
- The absolute value of the rounded difference is returned as the result.
Flowchart:
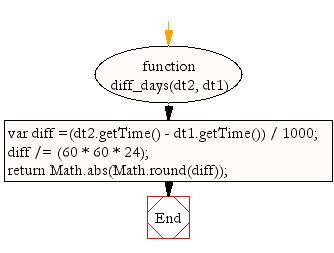
Live Demo:
See the Pen JavaScript - Get time differences in days between two dates-date-ex-46 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that computes the day difference between two dates by dividing the millisecond difference by 86400000.
- Write a JavaScript function that uses Math.round to adjust for partial days when computing the difference.
- Write a JavaScript function that returns a signed integer representing the day difference between two dates.
- Write a JavaScript function that validates date inputs and returns the day difference or an error if invalid.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get time differences in hours between two dates.
Next: Write a JavaScript function to get time differences in weeks between two dates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.