JavaScript: Calculate the product of values in an array
JavaScript Math: Exercise-18 with Solution
Product of Array Values
Write a JavaScript function to calculate the product of values in an array.
Test Data:
console.log(product([1,2,3]));
console.log(product([100,-200,3]));
console.log(product([1,2,'a',3]));
Output:
6
-60000
6
Visual Presentation:
Sample Solution:
JavaScript Code:
// Define a function named sum that calculates the sum of an array of numbers.
function sum(input){
// Check if the input is an array, if not, return false.
if (toString.call(input) !== "[object Array]")
return false;
var total = 0;
// Iterate through the input array and sum the numeric elements.
for(var i = 0; i < input.length; i++) {
// If the element is not a number, skip to the next element.
if(isNaN(input[i])){
continue;
}
// Add the numeric value of the element to the total.
total += Number(input[i]);
}
// Return the total sum.
return total;
}
// Output the sum of the numbers in the array [1, 2, 3] to the console.
console.log(sum([1, 2, 3]));
// Output the sum of the numbers in the array [100, -200, 3] to the console.
console.log(sum([100, -200, 3]));
// Output the sum of the numbers in the array [1, 2, 'a', 3] to the console.
console.log(sum([1, 2, 'a', 3]));
Output:
6 -60000 6
Flowchart:
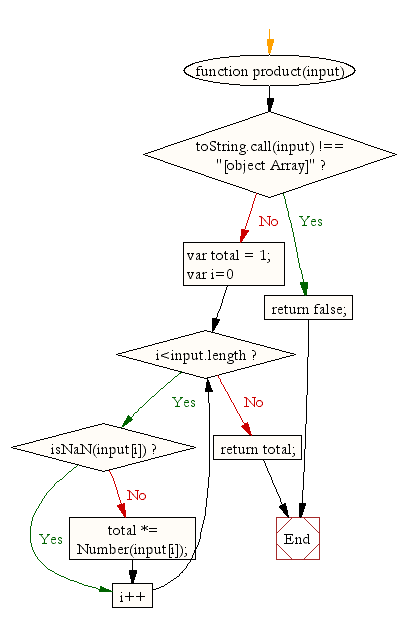
Live Demo:
See the Pen javascript-math-exercise-18 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates the product of all numeric values in an array, skipping non-numeric values.
- Write a JavaScript function that multiplies array elements using a for loop to obtain the cumulative product.
- Write a JavaScript function that computes the product using the reduce() method and manages empty arrays appropriately.
- Write a JavaScript function that recursively multiplies the elements of an array and handles cases where the product might be zero.
Go to:
PREV : Sum of Array Values.
NEXT : Pythagorean Theorem.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.