JavaScript: Currency math (add, subtract, multiply, division etc.)
JavaScript Math: Exercise-25 with Solution
Currency Math Operations
Write a JavaScript function to do currency math (add, subtract, multiply, divide etc.).
Test Data :
n1 = '$40.24', n2 = '$21.57';
Sample Solution:
JavaScript Code:
// Declare variables 'n1' and 'n2' and assign them string values representing dollar amounts.
var n1 = '$40.24';
var n2 = '$21.57';
// Define a regular expression pattern to match non-numeric characters.
var regp = /[^0-9.-]+/g;
// Remove non-numeric characters from 'n1' and 'n2', convert the resulting strings to floating-point numbers, and perform addition. Output the result to the console.
console.log(parseFloat(n1.replace(regp, '')) + parseFloat(n2.replace(regp, '')));
// Remove non-numeric characters from 'n1' and 'n2', convert the resulting strings to floating-point numbers, and perform subtraction. Output the result to the console.
console.log(parseFloat(n1.replace(regp, '')) - parseFloat(n2.replace(regp, '')));
// Remove non-numeric characters from 'n1' and 'n2', convert the resulting strings to floating-point numbers, and perform multiplication. Output the result to the console.
console.log(parseFloat(n1.replace(regp, '')) * parseFloat(n2.replace(regp, '')));
// Remove non-numeric characters from 'n1' and 'n2', convert the resulting strings to floating-point numbers, and perform division. Output the result to the console.
console.log(parseFloat(n1.replace(regp, '')) / parseFloat(n2.replace(regp, '')));
Output:
61.81 18.67 867.9768 1.865554010199351
Visual Presentation:
Flowchart:
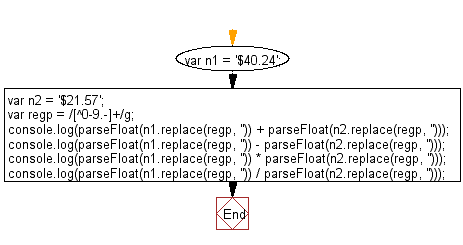
Live Demo:
See the Pen javascript-math-exercise-25 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that performs addition, subtraction, multiplication, and division on currency strings with proper decimal handling.
- Write a JavaScript function that converts currency-formatted strings to numbers, performs arithmetic operations, and returns a formatted currency string.
- Write a JavaScript function that simulates currency math by using integer arithmetic to avoid floating point precision errors.
- Write a JavaScript function that processes currency values, performs calculations, and consistently rounds the result to two decimal places.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to round a number to a specified number of digits and strip extra zeros (if any).
Next: Write a JavaScript function to calculate the nth root of a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.