JavaScript: Limit a value inside a certain range
JavaScript Math: Exercise-37 with Solution
Limit Value Within Range
Write a JavaScript function to limit a value inside a certain range.
Note : If the value is higher than max it will return max. and if the value is smaller than min it will return the min.
Test Data :
console.log(value_limit(7, 1, 12));
7
console.log(value_limit(-7, 0, 12));
0
console.log(value_limit(15, 0, 12));
12
Sample Solution:
JavaScript Code:
// Define a function named value_limit that restricts a value within a specified range.
function value_limit(val, min, max) {
// If val is less than min, return min; otherwise, if val is greater than max, return max; otherwise, return val.
return val < min ? min : (val > max ? max : val);
}
// Output the result of restricting the value 7 within the range [1, 12] to the console.
console.log(value_limit(7, 1, 12));
// Output the result of restricting the value -7 within the range [0, 12] to the console.
console.log(value_limit(-7, 0, 12));
// Output the result of restricting the value 15 within the range [0, 12] to the console.
console.log(value_limit(15, 0, 12));
Output:
7 0 12
Visual Presentation:
Flowchart:
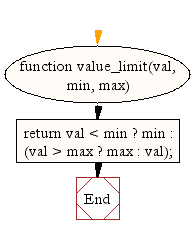
Live Demo:
See the Pen javascript-math-exercise-37 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that clamps a value within a specified minimum and maximum range using conditional operators.
- Write a JavaScript function that utilizes Math.min and Math.max to restrict a value between two boundaries.
- Write a JavaScript function that returns the original value if it is within range, or the nearest limit if it is out of range.
- Write a JavaScript function that implements range limiting using ternary operators without relying on built-in functions.
Go to:
PREV : Check If Number Is Power of Two.
NEXT : Check If Number Has Decimal.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.