JavaScript: Create random background color
JavaScript Math: Exercise-40 with Solution
Generate Random Background Color
Write a JavaScript function to create a random background color.
Visual Presentation:
Sample Solution:
JavaScript Code:
// Define a function named random_bg_color that generates a random RGB color and sets it as the background color of the document body.
function random_bg_color() {
// Generate random values for red, green, and blue components between 0 and 255.
var x = Math.floor(Math.random() * 256);
var y = Math.floor(Math.random() * 256);
var z = Math.floor(Math.random() * 256);
// Construct the RGB color string.
var bgColor = "rgb(" + x + "," + y + "," + z + ")";
// Output the generated color to the console.
console.log(bgColor);
// Set the background color of the document body to the generated color.
document.body.style.background = bgColor;
}
// Call the random_bg_color function to set a random background color.
random_bg_color();
Output:
rgb(251,34,92)
Flowchart:
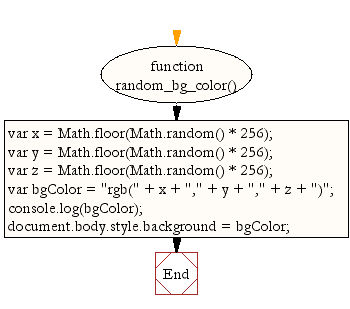
Live Demo:
See the Pen javascript-math-exercise-40 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that generates a random hexadecimal color code and applies it as the background color of the page.
- Write a JavaScript function that creates a random RGB color and assigns it as the background of a specific element.
- Write a JavaScript function that produces random pastel background colors and applies them to a series of elements.
- Write a JavaScript function that changes the background color to a random value at set intervals using setInterval.
Go to:
PREV : Format Integer with Thousands Separator.
NEXT : Count Integer Digits.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.