JavaScript: Count the digits of an integer
JavaScript Math: Exercise-41 with Solution
Count Integer Digits
Write a JavaScript function to count integer digits.
Sample Solution:
JavaScript Code:
// Define a function named digits_count that counts the number of digits in a given number.
function digits_count(n) {
// Initialize a variable to store the count of digits.
var count = 0;
// Check if the number is greater than or equal to 1, and if so, increment the count.
if (n >= 1) ++count;
// Repeat the following steps while the number divided by 10 is greater than or equal to 1.
while (n / 10 >= 1) {
// Divide the number by 10 to remove one digit.
n /= 10;
// Increment the count to keep track of the number of digits removed.
++count;
}
// Return the final count of digits.
return count;
}
// Output the result of counting the digits in the number 12112 to the console.
console.log(digits_count(12112));
// Output the result of counting the digits in the number 457 to the console.
console.log(digits_count(457));
Output:
5 3
Flowchart:
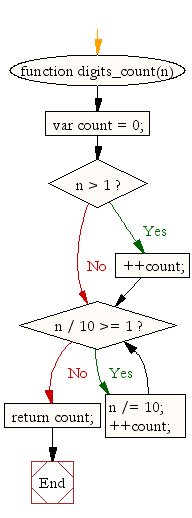
Live Demo:
See the Pen javascript-math-exercise-41 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that counts the number of digits in an integer by converting it to a string.
- Write a JavaScript function that uses iterative division to count the digits in an integer without string conversion.
- Write a JavaScript function that handles negative numbers by taking the absolute value before counting digits.
- Write a JavaScript function that calculates the number of digits using a while loop and division by 10.
Go to:
PREV : Generate Random Background Color.
NEXT : Calculate Combination (nCr).
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.