PHP Challenges: Check whether a sequence of numbers is an arithmetic progression or not
Write a PHP program to check whether a sequence of numbers is an arithmetic progression or not.
Input : array(5, 7, 9, 11)
In mathematics, an arithmetic progression or arithmetic sequence is a sequence of numbers such that the difference between the consecutive terms is constant.
For example, the sequence 5, 7, 9, 11, 13, 15 ... is an arithmetic progression with common difference of 2.
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to check if an array forms an arithmetic sequence
function is_arithmetic($arr)
{
// Calculate the common difference of the sequence
$delta = $arr[1] - $arr[0];
// Iterate through the array elements
for ($index = 0; $index < sizeof($arr) - 1; $index++)
{
// Check if the difference between consecutive elements is not equal to the common difference
if (($arr[$index + 1] - $arr[$index]) != $delta)
{
// If not, return "Not an arithmetic sequence"
return "Not an arithmetic sequence";
}
}
// If all differences are equal to the common difference, return "An arithmetic sequence"
return "An arithmetic sequence";
}
// Define two arrays
$my_arr1 = array(6, 7, 9, 11);
$my_arr2 = array(5, 7, 9, 11);
// Test the is_arithmetic function with the defined arrays and print the results
print_r(is_arithmetic($my_arr1) . "\n");
print_r(is_arithmetic($my_arr2) . "\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- Function definition:
- The code defines a function named "is_arithmetic()" which checks if an array forms an arithmetic sequence. It takes an array '$arr' as input.
- Inside the function:
- It calculates the common difference between consecutive elements of the array, assuming the first two elements represent an arithmetic sequence.
- It iterates through the array elements using a "for" loop.
- Within each iteration, it checks if the difference between consecutive elements is equal to the calculated common difference.
- If any difference is not equal to the common difference, it returns "Not an arithmetic sequence".
- If all differences are equal to the common difference, it returns "An arithmetic sequence".
- Function Call & Testing:
- Two arrays '$my_arr1' and '$my_arr2' are defined with different sets of numbers.
- The "is_arithmetic()" function is called twice with these arrays as input.
- Finally the "print_r()" function displays whether each input array forms an arithmetic sequence.
Sample Output:
Not an arithmetic sequence An arithmetic sequence
Flowchart:
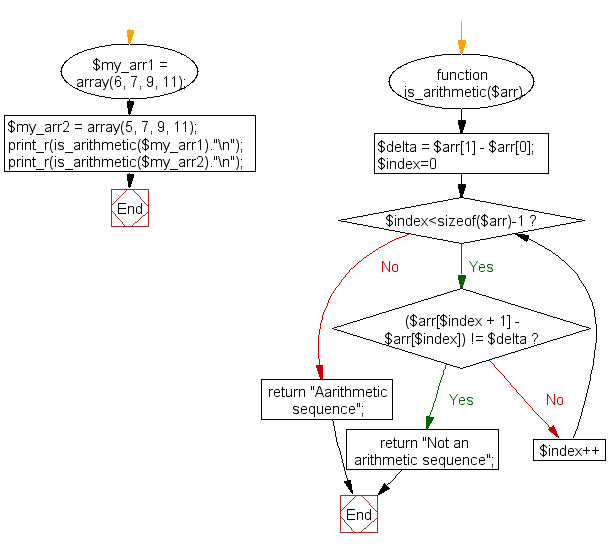
Go to:
PREV : Write a PHP program to reverse the bits of an integer (32 bits unsigned).
NEXT : Write a PHP program to check whether a sequence of numbers is a geometric progression or not.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.