PHP script: Display active session count on server
PHP Cookies and Sessions: Exercise-12 with Solution
Write a PHP script to display the number of active sessions on the server.
Sample Solution:
PHP Code :
<?php
// Set the session save path
session_save_path('i:/custom/');
// Get the session save path directory
$sessionSavePath = session_save_path();
// Get all session files in the save path directory
$sessionFiles = glob($sessionSavePath . '/*');
// Initialize the session counter
$activeSessions = 0;
// Iterate through the session files
foreach ($sessionFiles as $sessionFile) {
// Check if the session file is valid
if (is_file($sessionFile) && filectime($sessionFile) + ini_get('session.gc_maxlifetime') > time()) {
$activeSessions++;
}
}
echo "Number of active sessions: " . $activeSessions;
?>
Sample Output:
Number of active sessions: 0
Explanation:
In the above exercise -
- We set the session save path using session_save_path('i:/custom/').
- Retrieve the session save path using session_save_path() and store it in the variable $sessionSavePath.
- Use glob() to get an array of all session files in the session save path directory.
- Initialize the session counter variable $activeSessions to 0.
- Iterate through the session files using a foreach loop.
- For each session file, we check if it is a valid file and if its creation time plus the maximum session lifetime (configured by session.gc_maxlifetime) is greater than the current time. If both conditions are true, we increment the $activeSessions counter.
- Finally, display the number of active sessions using echo.
Flowchart:
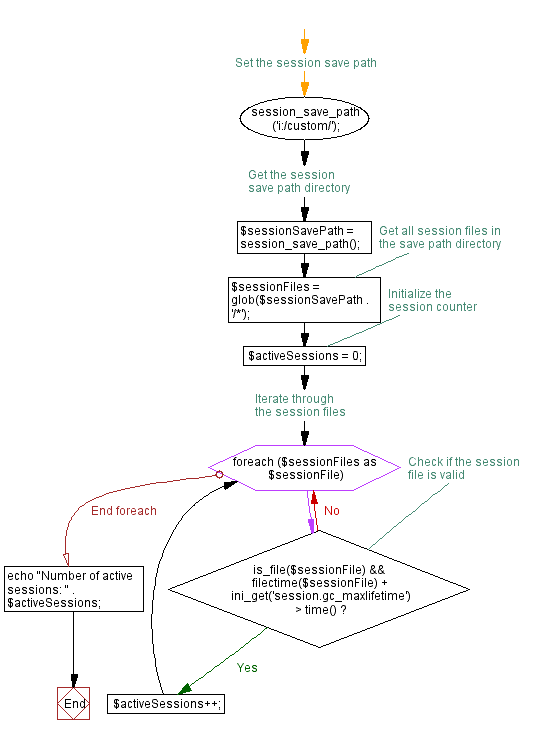
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Set PHP session timeout: 30 minutes of inactivity.
Next: PHP script: Limit maximum concurrent sessions to 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics