PHP File: Check if a file is writable or not
10. Check if File is Writable
Write a PHP program that checks if a file is writable and displays appropriate messages.
Sample Solution:
PHP Code :
<?php
$filename = "i:/test.txt";
try {
if (is_writable($filename)) {
echo "The file is writable.";
} else {
echo "The file is not writable.";
}
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
The file is writable.
Explanation:
In the above exercise -
- The $filename variable holds the name of the file you want to check.
- Inside the try block, we use the is_writable() function to check if the file is writable. If it is writable, we display the message "The file is writable." If it is not writable, we display the message "The file is not writable."
- If an error occurs during the check, the catch block will catch the exception and display an error message using $e-<getMessage().
Flowchart:
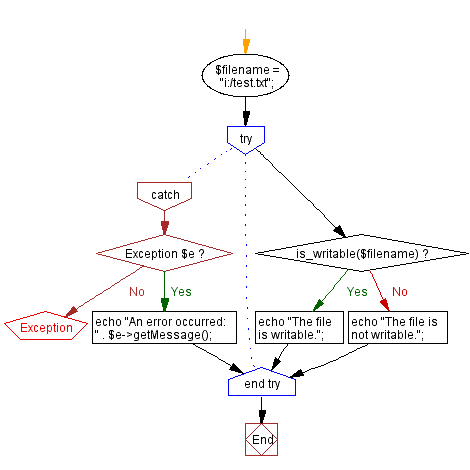
For more Practice: Solve these Related Problems:
- Write a PHP script to determine if a file is writable and if not, change its permissions programmatically.
- Write a PHP function that checks a list of files for writability and outputs which ones are not writable.
- Write a PHP program to attempt writing to a file and gracefully handle errors if the file is not writable.
- Write a PHP script to display a detailed message indicating the writable status of a file along with its permissions.
Go to:
PREV : Human-Readable File Size.
NEXT : Append a String to a File.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.