PHP Array Exercises : Get an array of all elements which have the keys that are present in another array
49. Get Array Entries with Specific Keys
Write a PHP script to get an array containing all the entries of an array which have the keys that are present in another array.
Sample Solution:
PHP Code:
<?php
// Original associative array
$first_array = array('c1' => 'Red', 'c2' => 'Green', 'c3' => 'White', 'c4' => 'Black');
// Array containing keys to check for intersection
$second_array = array('c2', 'c4');
// Use array_flip to swap keys and values, then apply array_intersect_key to find common keys
$result = array_intersect_key($first_array, array_flip($second_array));
// Display the result
print_r($result);
?>
Output:
Array ( [c2] => Green [c4] => Black )
Flowchart:
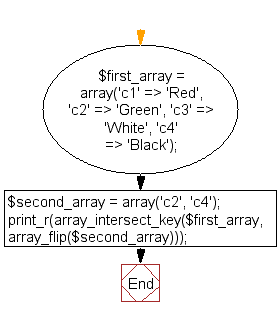
For more Practice: Solve these Related Problems:
- Write a PHP script to filter an associative array and return a new array containing only the entries whose keys are present in a second array.
- Write a PHP function to compare the keys of an array against a given list and output the matched key-value pairs.
- Write a PHP program to use array_intersect_key() to extract a subset of an array based on a provided keys array.
- Write a PHP script to manually loop through an associative array and build a new array from entries matching specified keys.
Go to:
PREV : Set Union of Two Arrays.
NEXT :Get Last Array Value Without Affecting Pointer.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.