PHP Date Exercises : Convert seconds into days, hours, minutes and seconds
21. Convert Seconds into Days, Hours, Minutes, Seconds
Write a PHP script to convert seconds into days, hours, minutes and seconds.
Sample seconds : 200000
Sample Solution:
PHP Code:
<?php
// Define a function to convert seconds into a human-readable format
function convert_seconds($seconds)
{
// Create DateTime objects representing the start and end timestamps
$dt1 = new DateTime("@0");
$dt2 = new DateTime("@$seconds");
// Calculate the difference between the two timestamps
$diff = $dt1->diff($dt2);
// Format the difference to display days, hours, minutes, and seconds
return $diff->format('%a days, %h hours, %i minutes and %s seconds');
}
// Call the function and echo the result
echo convert_seconds(200000)."\n";
?>
Output:
2 days, 7 hours, 33 minutes and 20 seconds
Explanation:
In the exercise above,
- function convert_seconds($seconds): Defines a function named convert_seconds that takes a number of seconds as input.
- $dt1 = new DateTime("@0");: Creates a DateTime object representing the start timestamp (epoch time).
- $dt2 = new DateTime("@$seconds");: Creates a DateTime object representing the end timestamp, calculated based on the provided number of seconds.
- $diff = $dt1->diff($dt2);: Calculates the difference between the two timestamps.
- return $diff->format('%a days, %h hours, %i minutes and %s seconds');: Formats the difference to display the number of days, hours, minutes, and seconds.
- echo convert_seconds(200000)."\n";: Calls the "convert_seconds()" function with 200000 seconds as input and echoes the result.
Flowchart :
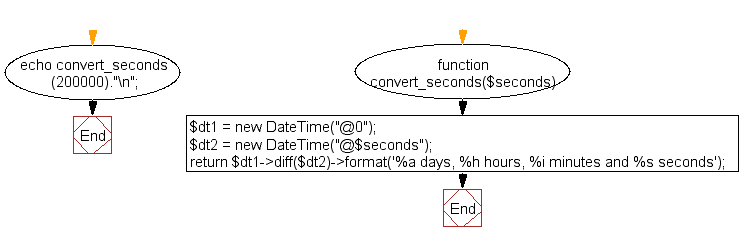
For more Practice: Solve these Related Problems:
- Write a PHP script to convert a given number of seconds into a formatted string displaying days, hours, minutes, and seconds.
- Write a PHP function that takes an integer number of seconds and returns an associative array with keys for days, hours, minutes, and seconds.
- Write a PHP program to convert seconds to time units and then output the result with proper pluralization of words.
- Write a PHP script to compute the time breakdown from seconds and format the output in a human-readable sentence.
Go to:
PREV : Get Previous Month Number.
NEXT : Last 6 Months from Current Month.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.