PHP for loop Exercises: Using nested for loop, construct a specific pattern
4. Construct Symmetric Star Pattern
Create a script to construct the following pattern, using a nested for loop.
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
Visual Presentation:
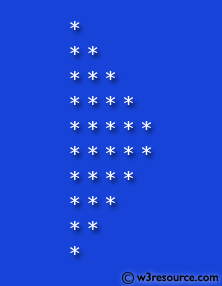
Sample Solution:
PHP Code:
<?php
// Define the number of rows
$n = 5;
// Loop to print the upper half of the diamond
for($i=1; $i<=$n; $i++)
{
// Loop to print the spaces and stars for each row
for($j=1; $j<=$i; $j++)
{
// Print a star surrounded by spaces
echo ' * ';
}
// Move to the next line after printing each row
echo '\n';
}
// Loop to print the lower half of the diamond
for($i=$n; $i>=1; $i--)
{
// Loop to print the spaces and stars for each row
for($j=1; $j<=$i; $j++)
{
// Print a star surrounded by spaces
echo ' * ';
}
// Move to the next line after printing each row
echo '\n ';
}
?>
Output:
* * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
Explanation:
In the exercise above,
- The code starts with a PHP opening tag <?php.
- It defines a variable '$n' and sets its value to 5, which represents the number of rows in the diamond pattern.
- The code then enters a loop to print the upper half of the diamond. This loop iterates from 1 to the value of '$n'.
- Inside the loop, there's another loop responsible for printing the spaces and stars for each row. It iterates from 1 to the current row number.
- Within this inner loop, a star surrounded by spaces (' * ') is echoed for each iteration.
- After printing all the spaces and stars for a row, a newline character (\n) is echoed to move to the next line.
- Once the upper half of the diamond is printed, the code enters another loop to print the lower half. This loop iterates from the value of '$n' down to 1.
- Inside this loop, the same process repeats: printing spaces and stars for each row, followed by moving to the next line.
- However, notice that extra space is printed after each line in the lower half of the diamond (echo '\n ';). This might be a typo or unintended behavior, as it seems to introduce additional space at the end of each line.
- Finally, PHP code ends with a closing tag ?>.
Flowchart :
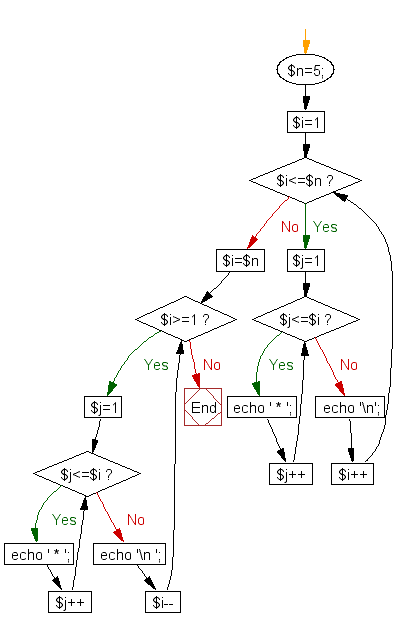
For more Practice: Solve these Related Problems:
- Write a PHP script to print a symmetric star pattern that increases to a maximum row and then decreases symmetrically using nested loops.
- Write a PHP function to generate a diamond-like star pattern where the middle row is repeated twice.
- Write a PHP program that uses nested loops to create an hourglass star pattern with a specified number of rows.
- Write a PHP script that constructs a mirror star pattern, ensuring the top and bottom halves are exact reflections.
Go to:
PREV : Construct Incremental Star Pattern.
NEXT : Factorial Calculation.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.