PHP Searching and Sorting Algorithm: Bubble sort
Write a PHP program to sort a list of elements using Bubble sort.
According to Wikipedia "Bubble sort, sometimes referred to as sinking sort, is a simple sorting algorithm that repeatedly steps through the list to be sorted, compares each pair of adjacent items and swaps them if they are in the wrong order. The pass through the list is repeated until no swaps are needed, which indicates that the list is sorted. The algorithm, which is a comparison sort, is named for the way smaller elements "bubble" to the top of the list. Although the algorithm is simple, it is too slow and impractical for most problems even when compared to insertion sort. It can be practical if the input is usually in sort order but may occasionally have some out-of-order elements nearly in position."
Step by step pictorial presentation :
Sample Solution :
PHP Code :
<?php
// Function to perform bubble sort on an array
function bubble_Sort($my_array )
{
// Loop until no swaps are made
do
{
$swapped = false; // Flag to track if any elements were swapped
// Iterate over the array
for( $i = 0, $c = count( $my_array ) - 1; $i < $c; $i++ )
{
// Compare adjacent elements
if( $my_array[$i] > $my_array[$i + 1] )
{
// Swap elements if they are in the wrong order
list( $my_array[$i + 1], $my_array[$i] ) =
array( $my_array[$i], $my_array[$i + 1] );
$swapped = true; // Set swapped flag to true
}
}
}
// Continue loop until no swaps are made
while( $swapped );
return $my_array; // Return the sorted array
}
// Test array
$test_array = array(3, 0, 2, 5, -1, 4, 1);
echo "Original Array :\n";
echo implode(', ',$test_array ); // Display original array
echo "\nSorted Array\n:";
echo implode(', ',bubble_Sort($test_array)). PHP_EOL; // Display sorted array
?>
Output:
Original Array : 3, 0, 2, 5, -1, 4, 1 Sorted Array : -1, 0, 1, 2, 3, 4, 5
Explanation:
In the exercise above,
- The code defines a function "bubble_Sort()" which takes an array '$my_array' as input and sorts it using the bubble sort algorithm.
- Inside the function, there is a do-while loop that continues until no swaps are made ($swapped flag remains false). This loop ensures that the sorting process continues until the array is fully sorted.
- Within the loop, there is a for loop that iterates over the array elements. It compares adjacent elements and swaps them if they are in the wrong order.
- The "list" construct is used for swapping the elements efficiently.
- If any elements are swapped during a pass through the array, the "$swapped" flag is set to true, indicating that the loop should continue.
- Once the loop completes without any swaps (i.e., the array is sorted), the function returns the sorted array.
- Finally, a test array '$test_array' is defined, and the original and sorted arrays are displayed using "implode()" function.
Flowchart :
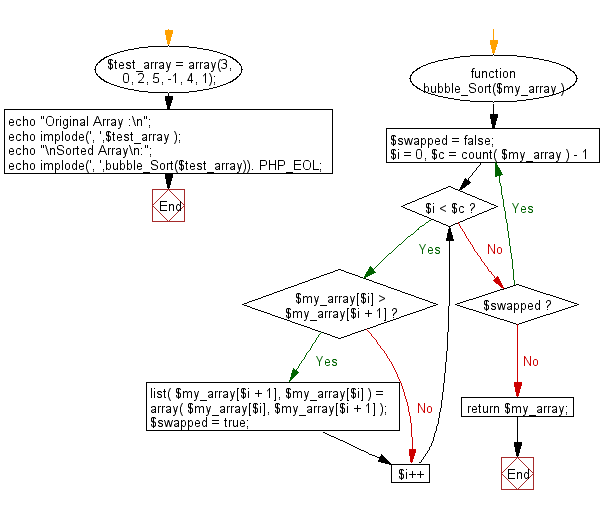
Go to:
PREV : Write a PHP program to sort a list of elements using Shell sort.
NEXT : Write a PHP program to sort a list of elements using Cocktail sort.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.