PHP Searching and Sorting Algorithm: Comb sort
Write a PHP program to sort a list of elements using Comb sort.
The Comb Sort is a variant of the Bubble Sort. Like the Shell sort, the Comb Sort increases the gap used in comparisons and exchanges. Some implementations use the insertion sort once the gap is less than a certain amount. The basic idea is to eliminate turtles, or small values near the end of the list, since in a bubble sort these slow the sorting down tremendously. Rabbits, large values around the beginning of the list do not pose a problem in bubble sort.
In bubble sort, when any two elements are compared, they always have a gap of 1. The basic idea of comb sort is that the gap can be much more than 1.
Visualization of comb sort :
Animation credits : Jerejesse
Sample Solution :
PHP Code :
<?php
// Function to perform Comb Sort on an array
function combSort($my_array){
$gap = count($my_array); // Set initial gap to the length of the array
$swap = true; // Flag to track if any swaps were made
while ($gap > 1 || $swap){ // Continue loop until gap is 1 and no swaps are made
if($gap > 1) $gap /= 1.25; // Reduce the gap by 1.25 if it's greater than 1
$swap = false; // Reset swap flag
$i = 0; // Initialize index variable
while($i+$gap < count($my_array)){ // Loop through the array
if($my_array[$i] > $my_array[$i+$gap]){ // Compare elements with the gap and swap if necessary
list($my_array[$i], $my_array[$i+$gap]) = array($my_array[$i+$gap],$my_array[$i]); // Swap elements
$swap = true; // Set swap flag to true
}
$i++; // Increment index
}
}
return $my_array; // Return the sorted array
}
$test_array = array(3, 0, 2, 5, -1, 4, 1); // Test array
echo "Original Array :\n";
echo implode(', ',$test_array ); // Display original array
echo "\nSorted Array\n:";
echo implode(', ',combSort($test_array)). PHP_EOL; // Display sorted array
?>
Output:
Original Array : 3, 0, 2, 5, -1, 4, 1 Sorted Array : -1, 0, 1, 2, 3, 4, 5
Explanation:
In the exercise above,
- combSort($my_array): This function implements the Comb Sort algorithm to sort an array.
- Algorithm Overview:
- Comb Sort is an extension of Bubble Sort and improves its performance by eliminating small values in the beginning of the array, which cause slow sorting in Bubble Sort.
- Variables:
- $gap: This variable represents the gap between the compared elements. It starts with the array length and decreases with each iteration until it becomes 1.
- $swap: This boolean flag indicates whether any swap operation has been performed during a pass through the array.
- Main Loop:
- The main loop continues until the gap becomes 1 and no swaps are performed. This loop iterates through the array multiple times, reducing the gap each time.
- Gap Reduction:
- Inside the loop, if the gap is greater than 1, it is reduced by a factor of 1.25 (a common choice for this algorithm).
- Array Traversal and Swapping:
- Another loop traverses the array while comparing elements separated by the current gap. If an element at position '$i' is greater than the element at position $i + $gap, they are swapped, and the swap flag is set to true.
- After completing the loop, the function returns the sorted array.
- Finally the code tests the function with a sample array '$test_array', prints the original array, sorts it using "combSort()", and prints the sorted array.
Flowchart :
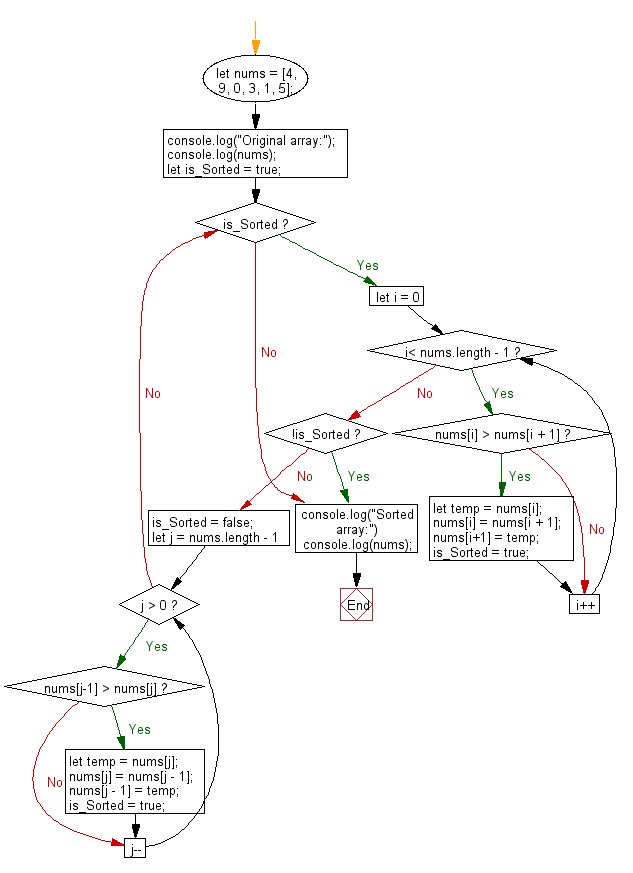
Go to:
PREV : Write a PHP program to sort a list of elements using Cocktail sort.
NEXT : Write a PHP program to sort a list of elements using Gnome sort.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.