Python Data Structure: Create a heapsort, pushing all values onto a heap and then popping off the smallest values one at a time
Python Data Structure: Exercise-9 with Solution
Write a Python program to create a heapsort, pushing all values onto a heap and then popping off the smallest values one at a time.
Sample Solution:
Python Code:
import heapq
def heapsort(h):
heap = []
for value in h:
heapq.heappush(heap, value)
return [heapq.heappop(heap) for i in range(len(heap))]
print(heapsort([10, 20, 50, 70, 90, 20, 50, 40, 60, 80, 100]))
Sample Output:
[10, 20, 20, 40, 50, 50, 60, 70, 80, 90, 100]
Flowchart:
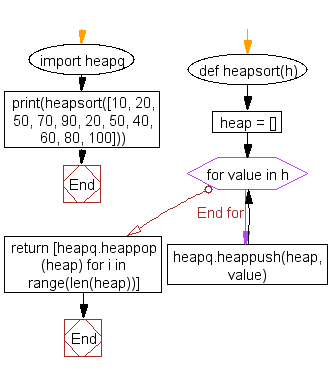
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to push an item on the heap, then pop and return the smallest item from the heap.
Next: Write a Python program to get the two largest and three smallest items from a dataset.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.