Python palindrome checker with Boolean logic
Python Boolean Data Type: Exercise-6 with Solution
Write a Python function that checks if a given string is a palindrome (reads the same backward as forward) using boolean values.
Sample Solution:
Code:
def is_palindrome(string):
text = ''.join(char.lower() for char in string if char.isalnum())
return text == text[::-1]
def main():
try:
input_string = input("Input a string: ")
if is_palindrome(input_string):
print("The string is a palindrome.")
else:
print("The string is not a palindrome.")
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Input a string: mamdam The string is not a palindrome.
Input a string: madam The string is a palindrome.
In the exercise above the program prompts the user to input a string, and then uses the "is_palindrome()" function to determine if the string is a palindrome. The string is converted to lowercase, non-alphanumeric characters are removed, and the cleaned string is checked for consistency when read backwards. It prints the appropriate message based on the results.
Flowchart:
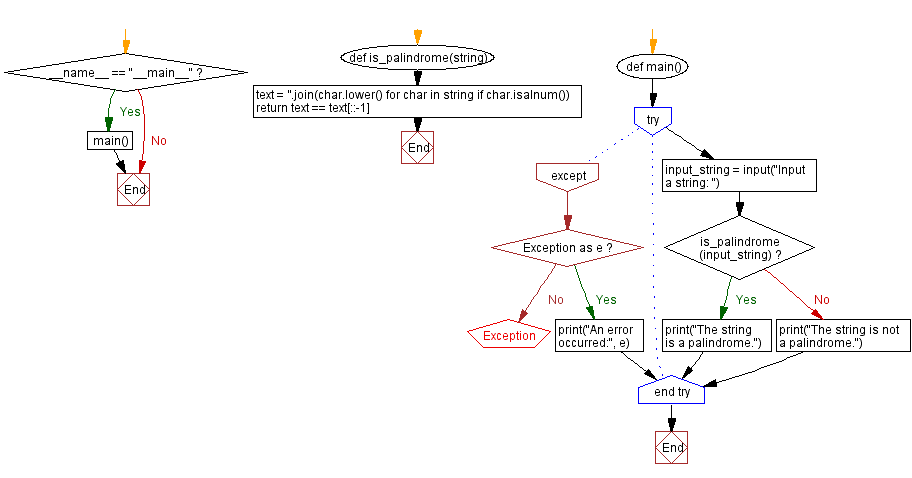
Previous:Python list empty check using Boolean logic.
Next: Python password validation with Boolean expressions.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics