Python function for word lengths OrderedDict
10. Words to Length Mapping
Write a Python function that takes a list of words and returns an OrderedDict where keys are the words and values are the lengths of the words.
Sample Solution:
Code:
from collections import OrderedDict
def word_lengths_ordered_dict(words):
ordered_dict = OrderedDict()
for word in words:
ordered_dict[word] = len(word)
return ordered_dict
def main():
words = ["Red", "Green", "Pink", "White", "Orange"]
result = word_lengths_ordered_dict(words)
for word, length in result.items():
print(f"{word}: {length}")
if __name__ == "__main__":
main()
Output:
Red: 3 Green: 5 Pink: 4 White: 5 Orange: 6
In the exercise above, the "word_lengths_ordered_dict()" function iterates through the list of words and adds each word as a key to the 'OrderedDict', with its corresponding length as the value. The "main()" function prints each word along with its length from the generated 'OrderedDict' using the "word_lengths_ordered_dict()" function.
Flowchart:
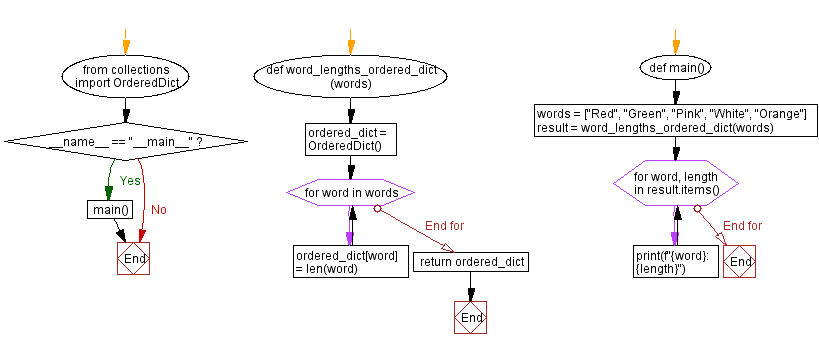
For more Practice: Solve these Related Problems:
- Write a Python function that takes a list of words and returns an OrderedDict where each key is a word and each value is its length, then print the OrderedDict.
- Write a Python script to build an OrderedDict from a list of words, sort the dictionary by the lengths of the words, and display the sorted dictionary.
- Write a Python program to create an OrderedDict mapping words to their lengths and then filter out words shorter than a given threshold.
- Write a Python function that takes a list of words, constructs an OrderedDict with words as keys and lengths as values, and then prints the dictionary with keys in the original order.
Go to:
Previous: Python generating random ASCII OrderedDict.
Next: Python Counter Exercises Home.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.