Python OrderedDict Key reordering
Python OrderedDict Data Type: Exercise-5 with Solution
Write a Python program to create an OrderedDict with the following key-value pairs:
'Laptop': 40
'Desktop': 45
'Mobile': 35
'Charger': 25
Now move the 'Desktop' key to the end of the dictionary and print the updated contents.
Sample Solution:
Code:
from collections import OrderedDict
# Create an OrderedDict with the given key-value pairs
ordered_dict = OrderedDict([
('Laptop', 40),
('Desktop', 45),
('Mobile', 35),
('Charger', 25)
])
print("Original OrderedDict:")
print(ordered_dict)
# Move the 'Desktop' key to the end
print("\nMove the 'Desktop' key to the end")
ordered_dict.move_to_end('Desktop')
# Print the updated OrderedDict
print("\nUpdated OrderedDict:")
print(ordered_dict)
Output:
Original OrderedDict: OrderedDict([('Laptop', 40), ('Desktop', 45), ('Mobile', 35), ('Charger', 25)]) Move the 'Desktop' key to the end Updated OrderedDict: OrderedDict([('Laptop', 40), ('Mobile', 35), ('Charger', 25), ('Desktop', 45)])
In this exercise above, the move_to_end() method of the OrderedDict is used to move the 'Desktop' key to the end of the dictionary, effectively changing its position within the dictionary.
Flowchart:
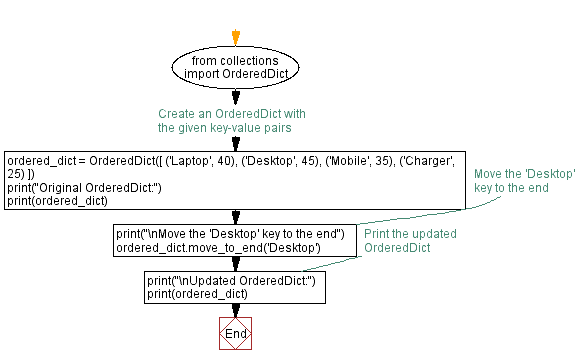
Previous: Python OrderedDict Reversal.
Next: Python OrderedDict Key-Value removal.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics