Python generating random ASCII OrderedDict
9. OrderedDict from Random Data
Write a Python program that creates an OrderedDict and populates it with random integer values as values and their ASCII characters as keys. Print the OrderedDict.
Sample Solution:
Code:
import random
from collections import OrderedDict
def random_ascii():
return chr(random.randint(65, 90))
def main():
ordered_dict = OrderedDict()
for _ in range(10):
key = random_ascii()
value = random.randint(1, 50)
ordered_dict[key] = value
print("OrderedDict:", ordered_dict)
if __name__ == "__main__":
main()
Output:
OrderedDict: OrderedDict([('Y', 43), ('B', 22), ('M', 9), ('D', 32), ('X', 27), ('N', 19), ('G', 19)])
In the exercise above, the "random_ascii()" function generates a random ASCII character using the "chr()" function and 'random.randint' to select a value within the ASCII range of uppercase letters (A-Z). The "main()" function creates an 'OrderedDict' and populates it with 10 random key-value pairs, where the key is a random ASCII character and the value is a random integer between 1 and 50. Finally, it prints the generated 'OrderedDict'.
Flowchart:
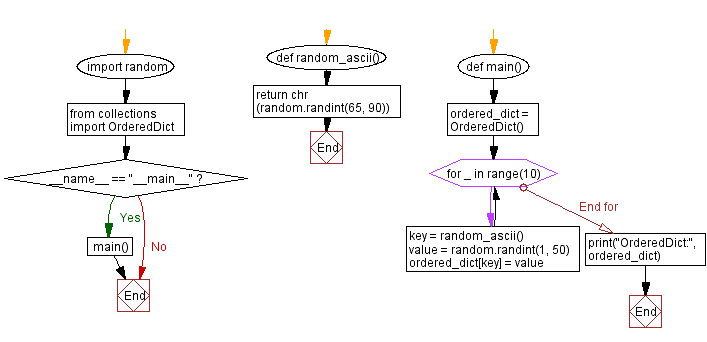
For more Practice: Solve these Related Problems:
- Write a Python program to generate a list of random integers, convert each integer to its corresponding ASCII character, and then create an OrderedDict mapping characters to integers.
- Write a Python script to create an OrderedDict with random integer values as keys (converted to characters) and then print the dictionary.
- Write a Python program to populate an OrderedDict with keys as random ASCII characters and values as random integers, and then display the OrderedDict in order.
- Write a Python function to create an OrderedDict from randomly generated data, ensuring that the keys are unique ASCII characters, and then print its contents.
Go to:
Previous: Python Removing key-Value pair from OrderedDict.
Next: Python function for word lengths OrderedDict.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.