NumPy: Concatenate two 2-dimensional arrays
Concatenate 2D Arrays
Write a NumPy program to concatenate two 2-dimensional arrays.
Sample arrays: ([[0, 1, 3], [5, 7, 9]], [[0, 2, 4], [6, 8, 10]]
Pictorial Presentation:
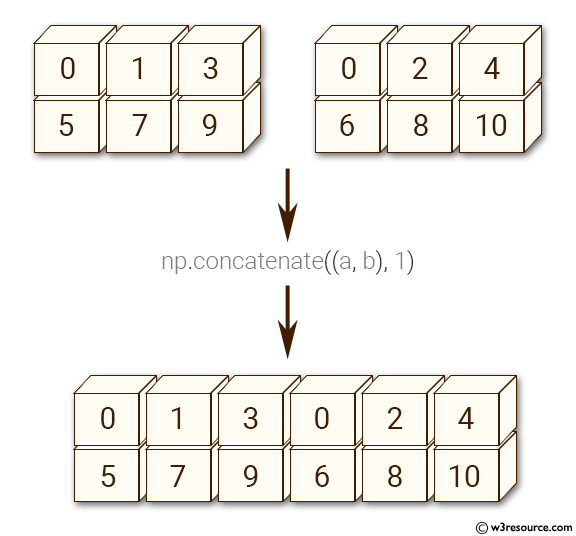
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating two NumPy arrays 'a' and 'b'
a = np.array([[0, 1, 3], [5, 7, 9]])
b = np.array([[0, 2, 4], [6, 8, 10]])
# Concatenating arrays 'a' and 'b' along the second axis (horizontally) using np.concatenate
c = np.concatenate((a, b), 1)
# Printing the concatenated array 'c'
print(c)
Sample Output:
[[ 0 1 3 0 2 4] [ 5 7 9 6 8 10]]
Explanation:
In the above code –
‘a = np.array([[0, 1, 3], [5, 7, 9]])’ creates a 2D array a with shape (2, 3).
‘b = np.array([[0, 2, 4], [6, 8, 10]])’ creates another 2D array b with shape (2, 3).
c = np.concatenate((a, b), 1): The np.concatenate() function is used to join the two arrays ‘a’ and ‘b’ along the second axis (axis=1). The resulting array ‘c’ has the shape (2, 6), with the columns of ‘b’ appended to the columns of ‘a’.
For more Practice: Solve these Related Problems:
- Write a NumPy program to horizontally concatenate two 2D arrays using np.concatenate and verify the combined shape.
- Implement vertical concatenation of two arrays and validate the resulting dimensions.
- Concatenate multiple 2D arrays along a new axis and then merge them back to a single array.
- Create a function that accepts a list of 2D arrays and concatenates them along a specified axis.
Go to:
PREV : Remove Single-Dimensional Entries
NEXT : Convert 1D Arrays to 2D (as Columns)
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.