NumPy: Create a vector of size 10 with values ranging from 0 to 1, both excluded
Vector of Size 10 (0–1 Excluded)
Write a NumPy program to create a vector of size 10 with values ranging from 0 to 1, both excluded.
Pictorial Presentation:
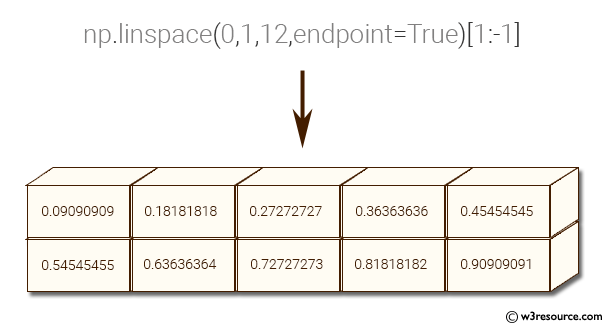
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating an array 'x' using NumPy's linspace function,
# generating 12 evenly spaced values between 0 and 1 (inclusive) with the 'endpoint' set to True,
# and selecting elements from index 1 to the second-to-last index using [1:-1]
x = np.linspace(0, 1, 12, endpoint=True)[1:-1]
# Printing the array 'x' that was created
print(x)
Sample Output:
[ 0.09090909 0.18181818 0.27272727 0.36363636 0.45454545 0.5454545 5 0.63636364 0.72727273 0.81818182 0.90909091]
Explanation:
In the above code –
x = np.linspace(0,1,12,endpoint=True): This line creates a 1D NumPy array with 12 evenly spaced values between 0 and 1 (both inclusive). The endpoint=True parameter ensures that the endpoint (1) is included in the array. The resulting array would look like this: [0., 0.09090909, 0.18181818, ..., 0.81818182, 0.90909091, 1.].
[1:-1]: This slice notation is applied to the array x to remove the first element (0.) and the last element (1.) of the array. The resulting array would look like this: [0.09090909, 0.18181818, ..., 0.81818182, 0.90909091].
print(x): This line prints the modified array after removing the first and the last elements: [0.09090909, 0.18181818, ..., 0.81818182, 0.90909091].
For more Practice: Solve these Related Problems:
- Write a NumPy program to create a vector of size 10 with values evenly spaced between 0 and 1, excluding the endpoints, using np.linspace.
- Implement a function that generates a vector with custom exclusion of boundary values and verifies its contents.
- Test the vector generation by ensuring that neither 0 nor 1 appears in the resulting array.
- Compare the outputs of np.linspace with and without endpoint exclusion to understand their differences.
Go to:
PREV : Check Values Present in Array
NEXT : Make Array Read-Only
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.