NumPy: Create a record array from a (flat) list of arrays
Create Record Array from Flat Lists
Write a NumPy program to create a record array from a (flat) list of arrays.
Sample arrays: [1,2,3,4], ['Red', 'Green', 'White', 'Orange'], [12.20,15,20,40]
Pictorial Presentation:
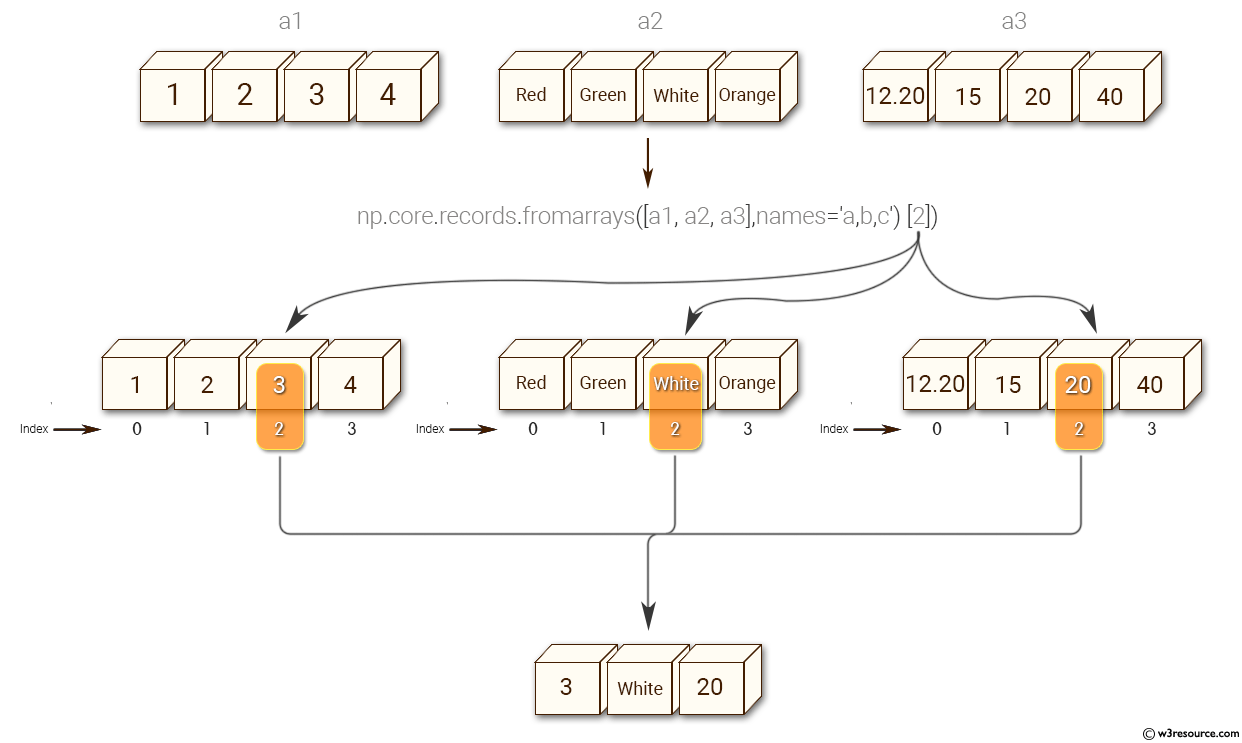
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating NumPy arrays 'a1', 'a2', and 'a3' containing different types of data
a1 = np.array([1, 2, 3, 4])
a2 = np.array(['Red', 'Green', 'White', 'Orange'])
a3 = np.array([12.20, 15, 20, 40])
# Creating a structured NumPy array 'result' using np.core.records.fromarrays
# The structured array contains fields 'a', 'b', and 'c' corresponding to arrays 'a1', 'a2', and 'a3' respectively
result = np.core.records.fromarrays([a1, a2, a3], names='a,b,c')
# Printing the first element of the structured array 'result'
print(result[0])
# Printing the second element of the structured array 'result'
print(result[1])
# Printing the third element of the structured array 'result'
print(result[2])
Sample Output:
(1, 'Red', 12.2) (2, 'Green', 15.0) (3, 'White', 20.0)
Explanation:
In the above exercise –
- a1=np.array([1,2,3,4]): Create a one-dimensional array a1 with integer values.
- a2=np.array(['Red','Green','White','Orange']): Create a one-dimensional array a2 with string values.
- a3=np.array([12.20,15,20,40]): Create a one-dimensional array a3 with floating-point values.
- result= np.core.records.fromarrays([a1, a2, a3],names='a,b,c'): Combine the three arrays into a single structured array using np.core.records.fromarrays(). The names parameter assigns field names 'a', 'b', and 'c' to the columns from arrays a1, a2, and a3, respectively.
- print(result[0]): Print the first record of the structured array.
- print(result[1]): Print the second record of the structured array.
- print(result[2]): Print the third record of the structured array.
For more Practice: Solve these Related Problems:
- Write a NumPy program to create a record array from multiple flat lists using np.rec.array.
- Construct a structured array from separate lists and verify the field names and data types.
- Create a record array from flat data and then access individual fields to ensure proper conversion.
- Implement a function that takes flat lists as input and returns a record array with correctly typed columns.
Go to:
PREV : Chunkify (3,4) Array Elements
NEXT : Generate 2D Gaussian-Like Array
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.