NumPy: Create a NumPy array of 10 integers from a generator
Generate 10 Integers from Generator
Write a NumPy program to create a NumPy array of 10 integers from a generator.
Pictorial Presentation:
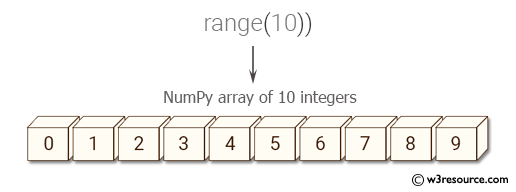
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a generator 'iterable' using a generator expression that yields values from 0 to 9
iterable = (x for x in range(10))
# Creating a NumPy array using np.fromiter() by converting the elements obtained from the iterable
# Specifying the data type as np.int to create an array of integers
print(np.fromiter(iterable, np.int))
Sample Output:
[0 1 2 3 4 5 6 7 8 9]
Explanation:
In the above code –
iterable = (x for x in range(10)): Define a generator expression iterable that generates numbers from 0 to 9 (10 is excluded). The generator expression is enclosed in parentheses and works by iterating through the range of numbers.
print(np.fromiter(iterable, np.int)): Call the np.fromiter() function to create a NumPy array from the iterable object. The second argument, np.int, specifies the desired data type for the elements in the resulting NumPy array. Finally, print the created NumPy array.
For more Practice: Solve these Related Problems:
- Write a NumPy program to generate 10 consecutive integers using a Python generator and convert them into an array.
- Create a function that yields integers from a generator and collects them into a NumPy array for validation.
- Compare the performance of a generator-based approach versus using np.arange for producing 10 integers.
- Test the generator output by converting it into an array and verifying the sequential order of the numbers.
Go to:
PREV : Suppress Scientific Notation in Array
NEXT : Add Extra Column to Array
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.