NumPy: How to add an extra column to a NumPy array
Add Extra Column to Array
Write a NumPy program to add an extra column to a NumPy array.
Pictorial Presentation:
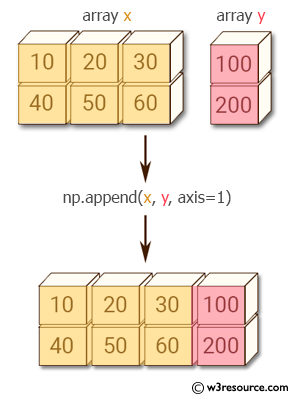
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a NumPy array 'x' with a shape of 2x3 (2 rows, 3 columns)
x = np.array([[10, 20, 30], [40, 50, 60]])
# Creating a NumPy array 'y' with a shape of 2x1 (2 rows, 1 column)
y = np.array([[100], [200]])
# Appending arrays 'x' and 'y' along the second axis (axis=1), concatenating them horizontally
# The resulting array will have a shape of 2x4 (2 rows, 4 columns)
print(np.append(x, y, axis=1))
Sample Output:
[[ 10 20 30 100] [ 40 50 60 200]]
Explanation:
‘x = np.array([[10,20,30], [40,50,60]])’ creates a 2x3 NumPy array 'x' with elements [[10, 20, 30], [40, 50, 60]].
‘y = np.array([[100], [200]])’ creates a 2x1 NumPy array 'y' with elements [[100], [200]].
print(np.append(x, y, axis=1)): Call the np.append() function to append the 'y' array to the 'x' array along the horizontal axis (axis=1). The resulting array will have the same number of rows as both 'x' and 'y', and its columns will be the combined columns of 'x' and 'y'.
For more Practice: Solve these Related Problems:
- Write a NumPy program to add an extra column to a 2D array using np.hstack and verify the updated shape.
- Create a function that appends a column vector to an existing matrix and checks for proper alignment.
- Use np.column_stack to merge a 1D array with a 2D array and validate the combined output.
- Test adding an extra column by concatenating a computed column (e.g., sum of rows) to the original array.
Go to:
PREV : Generate 10 Integers from Generator
NEXT : Find Distinct Rows in Array
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.