Python Exercise: Check a string represent an integer or not
35. String Represents Integer Checker
Write a Python program that checks whether a string represents an integer or not.
Pictorial Presentation:
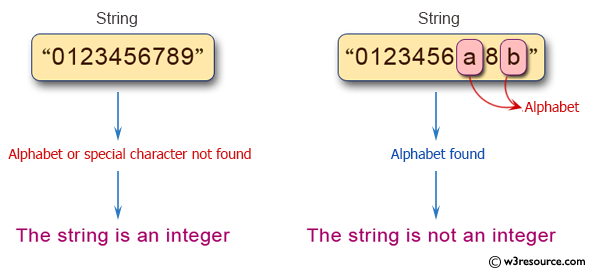
Sample Solution:
Python Code:
# Request input from the user to input a string and assign it to the variable 'text'
text = input("Input a string: ")
# Remove leading and trailing whitespaces from the input string using the 'strip()' function
text = text.strip()
# Check if the length of the cleaned text is less than 1
if len(text) < 1:
print("Input a valid text") # If the text length is less than 1, display a message asking for valid input
else:
# Check if all characters in the cleaned text are digits (0-9), indicating an integer
if all(text[i] in "0123456789" for i in range(len(text))):
print("The string is an integer.") # If all characters are digits, display a message indicating an integer
# Check if the text starts with a '+' or '-' and all subsequent characters are digits
elif (text[0] in "+-") and all(text[i] in "0123456789" for i in range(1, len(text))):
print("The string is an integer.") # If the conditions for a signed integer are met, display an integer message
else:
print("The string is not an integer.") # If none of the conditions are met, display a non-integer message
Sample Output:
Input a string: python The string is not an integer.
Flowchart :
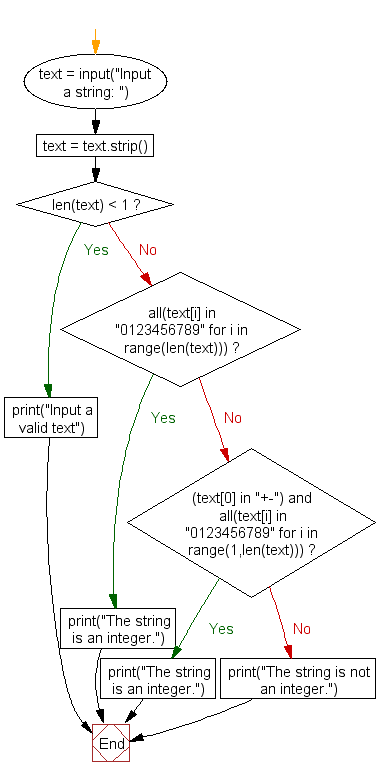
For more Practice: Solve these Related Problems:
- Write a Python program to check whether a given input string can be converted to an integer using exception handling.
- Write a Python program to validate if a string represents a valid integer using regular expressions.
- Write a Python program to determine if a string is an integer by checking if it consists solely of digits (with an optional leading sign).
- Write a Python program to implement a function that returns True if a string represents an integer and False otherwise.
Go to:
Previous: Write a Python program to sum of two given integers. However, if the sum is between 15 to 20 it will return 20.
Next: Write a Python program to check a triangle is equilateral, isosceles or scalene.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.