Python: Matches a string that has an 'a' followed by anything, ending in 'b'
9. a ... ending in b
Write a Python program that matches a string that has an 'a' followed by anything ending in 'b'.
Sample Solution:
Python Code:
import re
def text_match(text):
patterns = 'a.*?b$'
if re.search(patterns, text):
return 'Found a match!'
else:
return('Not matched!')
print(text_match("aabbbbd"))
print(text_match("aabAbbbc"))
print(text_match("accddbbjjjb"))
Sample Output:
Not matched! Not matched! Found a match!
Pictorial Presentation:
Flowchart:
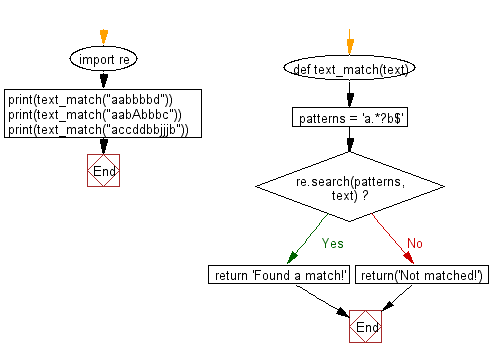
For more Practice: Solve these Related Problems:
- Write a Python program to match a string that starts with 'a' and ends with 'b', with any characters in between.
- Write a Python script to extract substrings that begin with 'a' and end with 'b' from a given text.
- Write a Python program to validate input strings that begin with 'a' and end with 'b', regardless of the middle content.
- Write a Python program to list all occurrences of substrings that start with 'a' and terminate with 'b' within a long string.
Go to:
Previous: Write a Python program to find sequences of one upper case letter followed by lower case letters.
Next: Write a Python program that matches a word at the beginning of a string.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.