Python Tkinter Spinbox: Custom range and step size made easy
Write a Python GUI program that creates a Spinbox widget with a custom range of values and step size using tkinter module.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
def update_value():
selected_value = spinbox_var.get()
result_label.config(text=f"Selected Value: {selected_value}")
parent = tk.Tk()
parent.title("Spinbox Example")
# Create a Spinbox widget with a custom range and step size
spinbox_var = tk.DoubleVar()
spinbox = ttk.Spinbox(parent, from_=0.0, to=10.0, increment=0.5, textvariable=spinbox_var)
spinbox.pack(padx=20, pady=20)
# Create a button to get the selected value
get_value_button = tk.Button(parent, text="Get Selected Value", command=update_value)
get_value_button.pack()
# Create a label to display the selected value
result_label = tk.Label(parent, text="", font=("Helvetica", 10))
result_label.pack()
parent.mainloop()
Explanation:
In the exercise above -
- Import 'tkinter' as 'tk' and 'ttk' for creating GUI components.
- Define a function "update_value()" that retrieves the selected value from the Spinbox widget and updates a label to display it.
- Create the main window using "tk.Tk()" and set its title.
- Creating a DoubleVar named 'spinbox_var' to hold the Spinbox value.
- Create a Spinbox widget named spinbox with a custom range from 0.0 to 10.0 and a step size of 0.5. We associate it with spinbox_var using the textvariable parameter.
- Create a button named 'get_value_button' that, when clicked, calls the "update_value()" function to retrieve and display the selected value.
- Create a label named 'result_label' that displays the selected value.
- The main event loop, root.mainloop(), starts the Tkinter application.
Sample Output:
Flowchart:
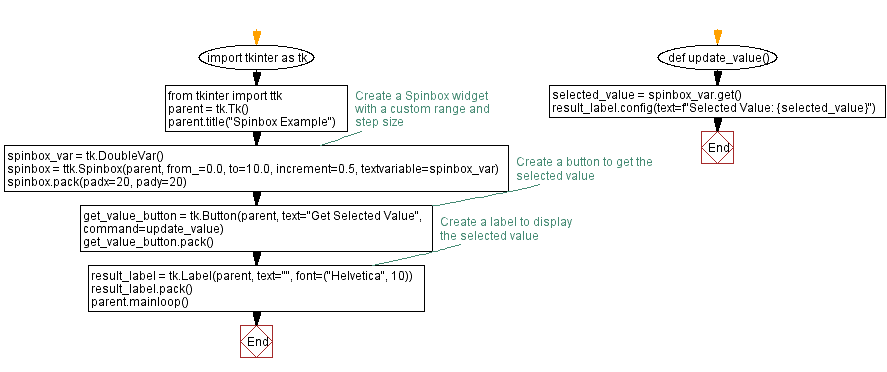
Go to:
Previous: Dynamic percentage updates.
Next: Multi-line text with word wrapping made easy.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.