C Exercises: Sort elements of an array in descending order
12. Sort Array Descending
Write a program in C to sort the elements of the array in descending order.
The task is to write a C program that sorts an array of integers in descending order. The program should prompt the user to input the size of the array and then the array elements. Finally, it should display the array elements sorted in descending order.
Visual Presentation:
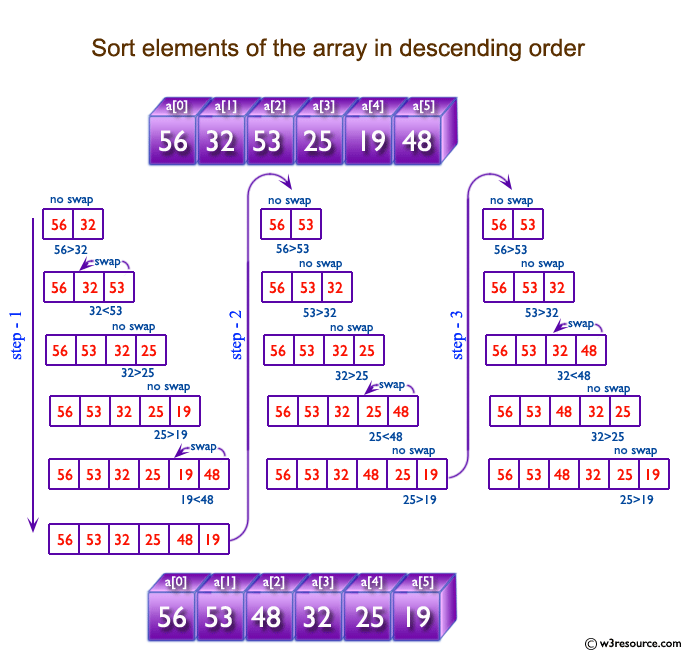
Sample Solution:
C Code:
#include <stdio.h>
void main()
{
int arr1[100];
int n, i, j, tmp;
// Prompt user for input
printf("\n\nSort elements of array in descending order:\n");
printf("----------------------------------------------\n");
printf("Input the size of array : ");
scanf("%d", &n);
// Input elements for the array
printf("Input %d elements in the array :\n", n);
for (i = 0; i < n; i++)
{
printf("element - %d : ", i);
scanf("%d", &arr1[i]);
}
// Sorting elements in descending order using the Bubble Sort algorithm
for (i = 0; i < n; i++)
{
for (j = i + 1; j < n; j++)
{
if (arr1[i] < arr1[j])
{
// Swap elements if they are in the wrong order
tmp = arr1[i];
arr1[i] = arr1[j];
arr1[j] = tmp;
}
}
}
// Print sorted elements in descending order
printf("\nElements of array are sorted in descending order:\n");
for (i = 0; i < n; i++)
{
printf("%d ", arr1[i]);
}
printf("\n\n");
}
Sample Output:
sort elements of array in descending order : ---------------------------------------------- Input the size of array : 3 Input 3 elements in the array : element - 0 : 5 element - 1 : 9 element - 2 : 1 Elements of array is sorted in descending order: 9 5 1
Flowchart:
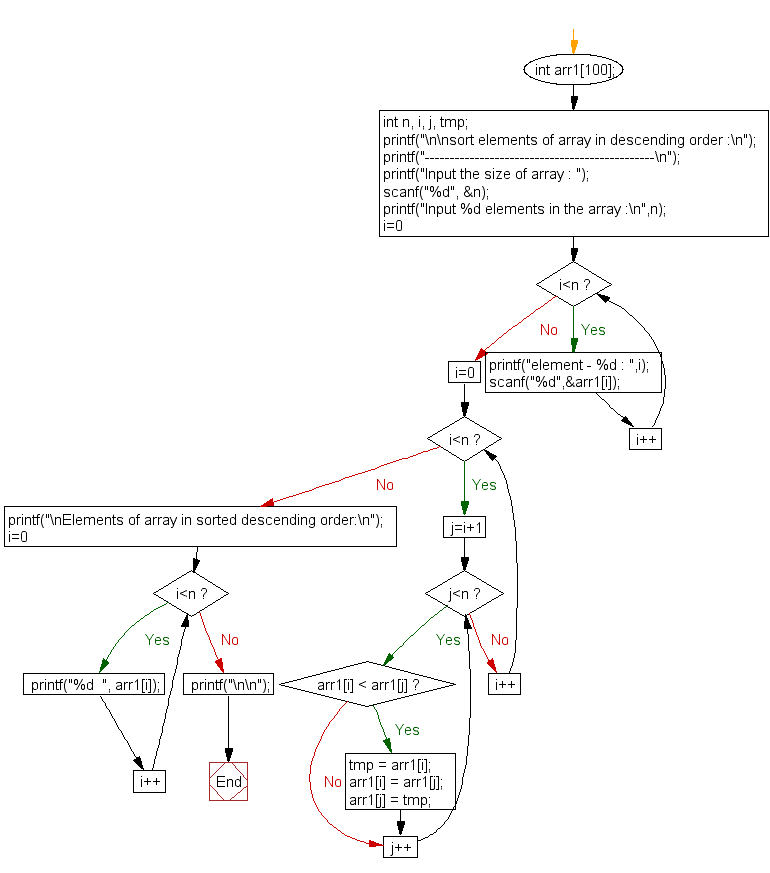
For more Practice: Solve these Related Problems:
- Write a C program to sort an array in descending order using quicksort.
- Write a C program to sort an array in descending order using insertion sort and pointer arithmetic.
- Write a C program to sort an array in descending order recursively.
- Write a C program to sort an array in descending order and then display the top k elements.
C Programming Code Editor:
Previous: Write a program in C to sort elements of array in ascending order.
Next: Write a program in C to insert New value in the array (sorted list ).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.