C Exercises: Read n number of values in an array and display it in reverse order
2. Array Reverse Display
Write a program in C to read n number of values in an array and display them in reverse order.
This task requires writing a C program to read a user-defined number of integer values into an array and then display these values in reverse order. After storing the values, the program should first print them in the original order and then print them in the reversed order.
Visual Presentation:
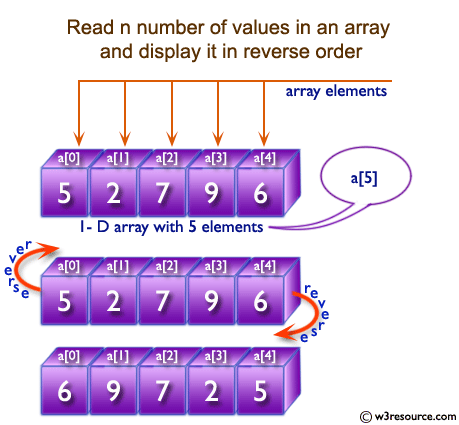
Pseudo code:
Start Declare n, i as integer variables Declare nums as an integer array of size n Read n from the user Read values into the array nums from the user in a loop from i=0 to n-1 Print "Array in reverse order: " For i=n-1 to 0 Step -1 Print nums [i] End For End
Sample Solution:
C Code:
#include <stdio.h>
// Main function
int main()
{
int i, n, a[100];
// Display a message to the user about the program's purpose
printf("\n\nRead n number of values in an array and display it in reverse order:\n");
printf("------------------------------------------------------------------------\n");
// Prompt the user to input the number of elements to store in the array
printf("Input the number of elements to store in the array :");
scanf("%d", &n);
// Prompt the user to input n elements into the array
printf("Input %d number of elements in the array :\n", n);
for (i = 0; i < n; i++)
{
printf("element - %d : ", i);
scanf("%d", &a[i]); // Read the input and store it in the array
}
// Display the values stored in the array
printf("\nThe values stored in the array are : \n");
for (i = 0; i < n; i++)
{
printf("% 5d", a[i]); // Print each element in the array
}
// Display the values stored in the array in reverse order
printf("\n\nThe values stored in the array in reverse are :\n");
for (i = n - 1; i >= 0; i--)
{
printf("% 5d", a[i]); // Print each element in reverse order
}
printf("\n\n");
return 0;
}
Sample Output:
Read n number of values in an array and display it in reverse order: ------------------------------------------------------------------------ Input the number of elements to store in the array :3 Input 3 number of elements in the array : element - 0 : 2 element - 1 : 5 element - 2 : 7 The values store into the array are : 2 5 7 The values store into the array in reverse are : 7 5 2
Explanation:
printf("Input the number of elements to store in the array :"); scanf("%d",&n); printf("Input %d number of elements in the array :\n",n); for(i=0;i<n;i++) { printf("element - %d : ",i); scanf("%d",&a[i]); } printf("\nThe values store into the array are : \n"); for(i=0;i<n;i++) { printf("% 5d",a[i]); } printf("\n\nThe values store into the array in reverse are :\n"); for(i=n-1;i>=0;i--) { printf("% 5d",a[i]); } printf("\n\n");
In the above code -
- The first printf statement prompts the user to input the number of elements they want to store in the array and stores it in the variable n using scanf.
- The second printf statement asks the user to input n number of elements into the array using a for loop, and stores each input in the corresponding index of the array a[i].
- The third printf statement then prints out the contents of the array in order using another for loop.
- The fourth printf statement then prints out the contents of the array in reverse order using yet another for loop, which iterates over the elements of a starting from the last element and printing each element out in reverse order.
Flowchart:
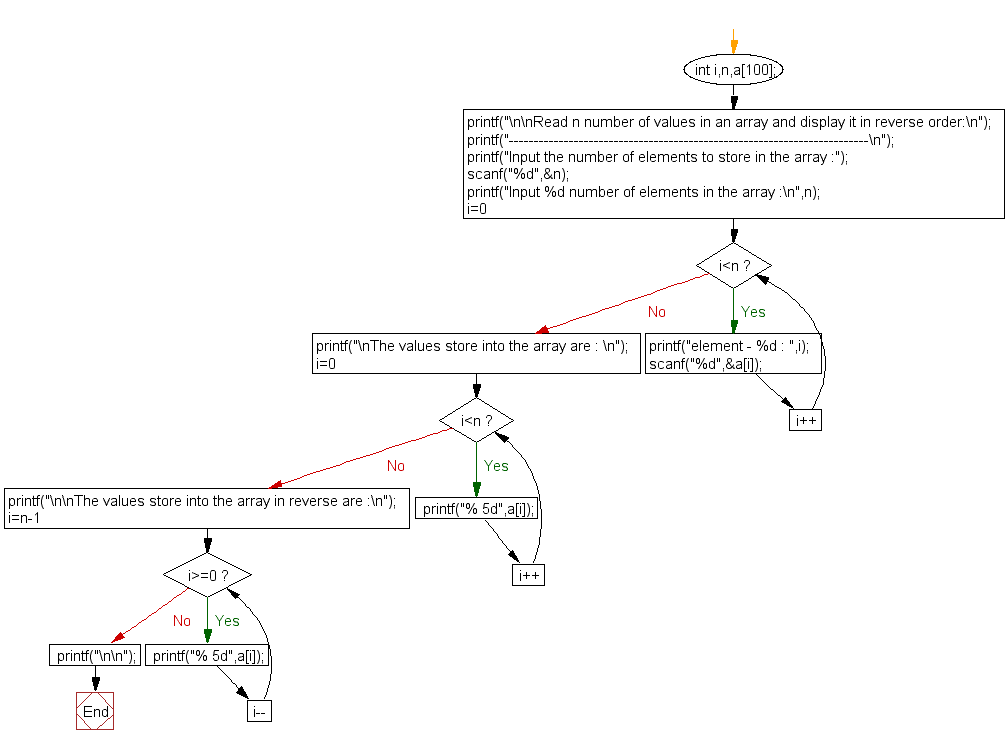
For more Practice: Solve these Related Problems:
- Write a C program to read n elements into an array and print them in alternating order from both ends.
- Write a C program to store numbers in an array and display them in reverse order using pointer decrement.
- Write a C program to input n numbers and then display the reverse order without using an auxiliary array.
- Write a C program to recursively reverse the contents of an array and print the reversed array.
C Programming Code Editor:
Previous: Write a program in C to store elements in an array and print it.
Next: Write a program in C to find the sum of all elements of an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.