C Exercises: Check whether an array is subset of another array
55. Check Subset of Another Array
Write a program in C to check whether an array is a subset of another array.
Expected Output :
The given first array is : 4 8 7 11 6 9 5 0 2
The given second array is : 5 4 2 0 6
The second array is the subset of first array.
To check if one array is a subset of another array, the program needs to verify that all elements of the second array are present in the first array. This can be done by iterating through each element of the second array and checking if it exists in the first array. If every element in the second array is found within the first array, then the second array is a subset of the first array.
Visual Presentation:
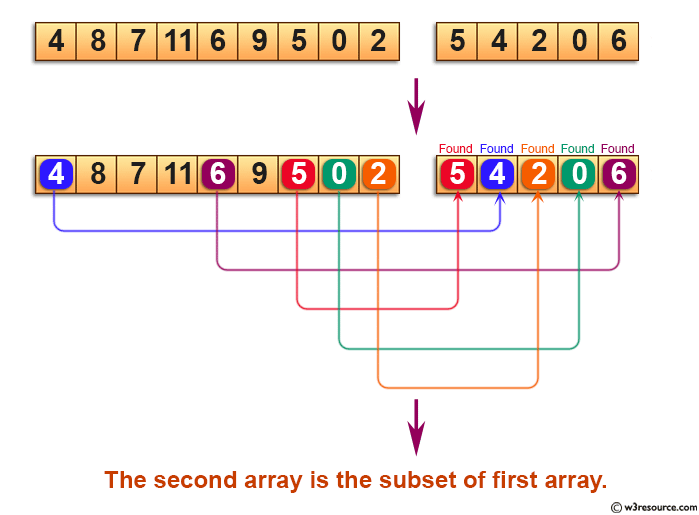
Sample Solution:
C Code:
#include <stdio.h>
// Function to check if arr2[] is a subset of arr1[]
int chkSubsetArray(int *arr1 , int arr1_size, int *arr2, int arr2_size) {
int i, j;
// Iterate through elements of arr2[]
for (i = 0; i < arr2_size; i++) {
// Search for current arr2[] element in arr1[]
for (j = 0; j < arr1_size; j++) {
// If element found in arr1[], break the loop
if (arr2[i] == arr1[j])
break;
}
// If the loop finishes without finding an element, arr2[] is not a subset
if (j == arr1_size)
return 0; // Not a subset
}
return 1; // arr2[] is a subset of arr1[]
}
int main() {
int arr1[] = {4, 8, 7, 11, 6, 9, 5, 0, 2};
int arr2[] = {5, 4, 2, 0, 6};
int n1 = sizeof(arr1) / sizeof(arr1[0]);
int n2 = sizeof(arr2) / sizeof(arr2[0]);
int i;
//------------- print first array ------------------
printf("The given first array is: ");
for (i = 0; i < n1; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
//------------- print second array ------------------
printf("The given second array is: ");
for (i = 0; i < n2; i++) {
printf("%d ", arr2[i]);
}
printf("\n");
// Check if arr2[] is a subset of arr1[]
if (chkSubsetArray(arr1, n1, arr2, n2))
printf("The second array is a subset of the first array.\n");
else
printf("The second array is not a subset of the first array.\n");
return 0;
}
Output:
The given first array is : 4 8 7 11 6 9 5 0 2 The given second array is : 5 4 2 0 6 The second array is the subset of first array.
Flowchart:/p>
For more Practice: Solve these Related Problems:
- Write a C program to check if one array is a subset of another by sorting both arrays first.
- Write a C program to determine if an array is a subset of another using hash tables.
- Write a C program to check subset relation by iterating through each element and using binary search.
- Write a C program to verify if one array is a subset of another and then display the common elements.
C Programming Code Editor:
Previous: Write a program in C to sort an array of 0s, 1s and 2s.
Next: Write a program in C to return the minimum number of jumps to reach the end of the array..
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.