C Exercises: Sum values before, after the maximum value in a sequence
C Basic-II: Exercise-5 with Solution
Write a C program that accepts a sequence of different values and calculates the sum of the values before and after the maximum value.
The sum of the values before the maximum value is 0, if there are no values before the maximum. Similarly, the sum of the values after the maximum value is 0, if there are no values after the maximum.
Sample Date:
1 2 3 -> 3 0
1 2 9 4 5 -> 3 9
2 2 2 2 -> 0 6
C Code:
#include <stdio.h> // Include standard input/output library
int N; // Declare a variable 'N' to hold the number of integers
int running_sum[101]; // Declare an array 'running_sum' to store cumulative sums
int main()
{
int max_x, max_y, i, x, d; // Declare variables for maximum, iteration, input, and cumulative sum
int sum_before, sum_after; // Declare variables for sum before and after maximum value
// Prompt the user for the number of integers in the sequence
printf("Number of integers want to input in the first sequence: ");
// Read the input and store it in 'N'
scanf("%d", &N);
// Prompt the user to input numbers for the sequence
printf("Input the numbers:\n");
max_x = 0; // Initialize 'max_x' to 0 for comparison
d = 0; // Initialize 'd' to 0 for cumulative sum
// Loop to read integers, calculate cumulative sum, and find maximum
for (i = 0; i< N; i++)
{
// Read an integer from the user
scanf("%d", &x);
d += x; // Update cumulative sum 'd'
running_sum[i] = d; // Store cumulative sum in 'running_sum' array
// Check if the current input is greater than current maximum
if (x >max_x)
{
max_x = x; // Update maximum value
max_y = i; // Store index of maximum value
}
}
// Calculate sum before maximum value
sum_before = running_sum[max_y] - max_x;
// Calculate sum after maximum value
sum_after = running_sum[N - 1] - max_x - sum_before;
// Print the sum before maximum value
printf("Sum before maximum value: %d\n", sum_before);
// Print the sum after maximum value
printf("Sum after maximum value: %d\n", sum_after);
// Return 0 to indicate successful program execution
return(0);
}
Sample Output:
Number of integers want to input in the first sequence: 5 Input the numbers: 1 2 9 4 5 Sum before maximum value: 3 Sum after maximum value: 9
Flowchart:
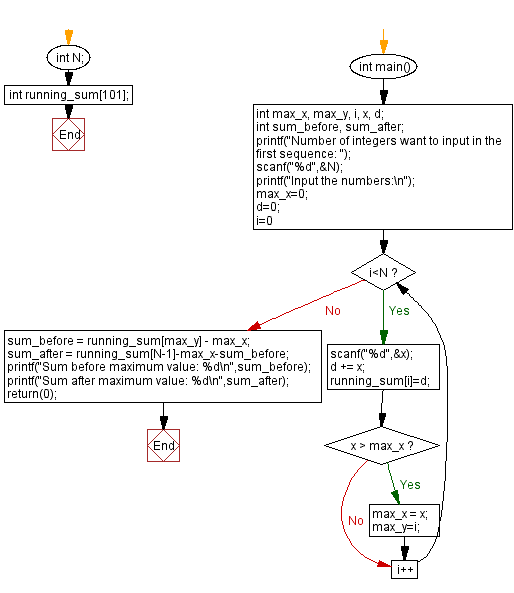
C Programming Code Editor:
Previous C Programming Exercise: Common element(s) of two sequences.
Next C Programming Exercise: Length of longest ascending contiguous subsequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-2/c-programming-basic-2-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics