C Exercises: Create an array taking two middle elements from a given array of integers of length even
C-programming basic algorithm: Exercise-46 with Solution
Write a C program to create an array taking two middle elements from a given array of integers of length even.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'print_array'
void print_array(int parray[], int size);
int main(void){
// Declaration and initialization of variables
int new_arr_size = 2; // Size of the new array
int arr_size; // Size of the original array
// Declaration and initialization of the original array 'nums1'
int nums1[] = { 1, 5, 7, 9, 11, 13};
// Calculating the size of the original array
arr_size = sizeof(nums1)/sizeof(nums1[0]);
// Printing elements in the original array
printf("Elements in original array are: ");
print_array(nums1, arr_size);
// Creating a new array with middle two elements of the original array
int result[] = { nums1[arr_size / 2 - 1], nums1[arr_size / 2] };
// Printing elements in the new array
printf("New array: ");
print_array(result, new_arr_size);
}
// Definition of the 'print_array' function
void print_array(int parray[], int size)
{
int i;
for( i=0; i<size-1; i++)
{
// Printing each element with a comma and a space
printf("%d, ", parray[i]);
}
// Printing the last element without a comma and space
printf("%d ", parray[i]);
// Printing a new line to separate the elements
printf("\n");
}
Sample Output:
Elements in original array are: 1, 5, 7, 9, 11, 13 New array: 7, 9
Pictorial Presentation:
Flowchart:
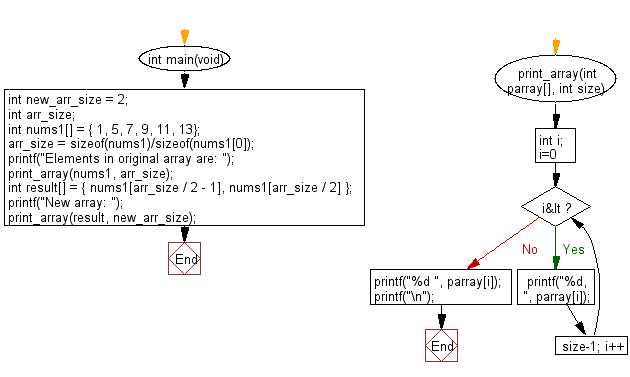
C Programming Code Editor:
Previous: Write a C program to compute the sum of the two given arrays of integers, length 3 and find the array which has the largest sum.
Next: Write a C program to create a new array from two give array of integers , each length 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics