C Exercises: Read a floating-point number and find the range where it belongs from four given ranges
Find range of a number from four given ranges
There are three given ranges. Write a C program that reads a floating-point number and finds the range where it belongs from four given ranges.
Ranges: 0-30, 31-50, 51-80, 81-100
Sample Solution:
C Code:
#include <stdio.h>
int main ()
{
// Declare a variable "x" to hold the input number
float x = 0;
// Prompt the user to input a number
printf("Input a number: ");
// Read the input number
scanf("%f", &x);
// Check the range of the input number and print the corresponding message
if (x >= 0 && x <= 30)
printf("Range [0,30]\n");
else if (x > 30 && x <= 50)
printf("Range (30,50]\n");
else if (x > 50 && x <= 80)
printf("Range (50,80]\n");
else if (x > 80 && x <= 100)
printf("Range (80,100]\n");
else
printf("\nNot within range..!\n");
}
Sample Output:
Input a number: 87 Range (80,100]
Flowchart:
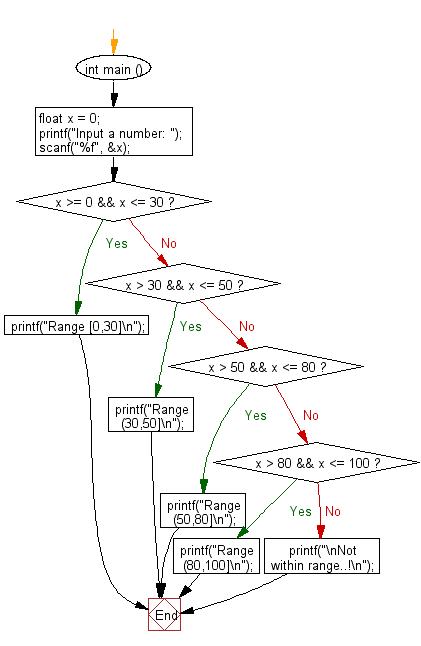
For more Practice: Solve these Related Problems:
- Write a C program to determine the appropriate range for a floating-point number using nested if-else statements.
- Write a C program to map a given float to one of four ranges by computing an index from an array of boundaries.
- Write a C program that uses a switch-case structure to output a range label for a user-supplied floating-point number.
- Write a C program to classify a float into one of four predefined ranges and display a custom message for each.
C programming Code Editor:
Previous: Write a C program to convert a currency value (floating point with two decimal places) to possible number of notes and coins.
Next: Write a C program that reads three integers and sort the numbers in ascending order. Print the original numbers and sorted numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.