C Exercises: Find the odd, even, positive and negative number form a given number and print a message
Classify a number as positive/negative and odd/even, or print "Zero"
Write a C program to find the odd, even, positive and negative numbers from a given number (integer) and print a message 'Number is positive odd' or 'Number is negative odd' or 'Number is positive even' or 'Number is negative even'. If the number is 0 print "Zero".
Sample Solution:
C Code:
#include <stdio.h>
int main () {
int b;
// Prompt the user to input a number (integer)
printf("Input a number (integer):\n");
// Read the input number
scanf("%d", &b);
// Check if the number is positive and even
if ((b % 2 == 0) && b > 0){
printf("Number is positive-even\n");
}
else{
// Check if the number is negative and even
if ((b % 2 == 0) && b < 0){
printf("Number is negative-even'\n");
}
else{
// Check if the number is positive and odd
if ((b % 2 !=0) && b > 0){
printf("Number is positive-odd\n");
}
else{
// Check if the number is negative and odd
if ((b % 2 != 0) && b < 0){
printf("Number is negative-odd\n");
}
else{
// If none of the above conditions are met, it must be zero
printf("Zero\n");
}
}
}
}
}
Sample Output:
Input a number (integer): 12 Number is positive-even
Flowchart:
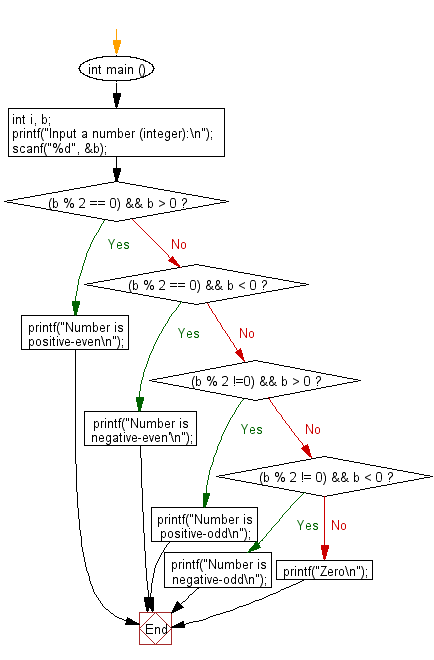
For more Practice: Solve these Related Problems:
- Write a C program to classify an integer into positive/negative and odd/even categories using nested if-else statements.
- Write a C program that uses a switch-case mechanism to display the classification of a number, including handling zero separately.
- Write a C program to determine the classification of a number with error-checking for non-integer input.
- Write a C program to use bitwise operators and conditional statements to classify a number’s sign and parity.
C programming Code Editor:
Previous: Write a C program to find and print the square of each even and odd values between 1 and a given number (4 < n < 101).
Next: Write a C program that accepts an integer from the user and divide all numbers between 1 and 100. Print those numbers where remainder value is 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.